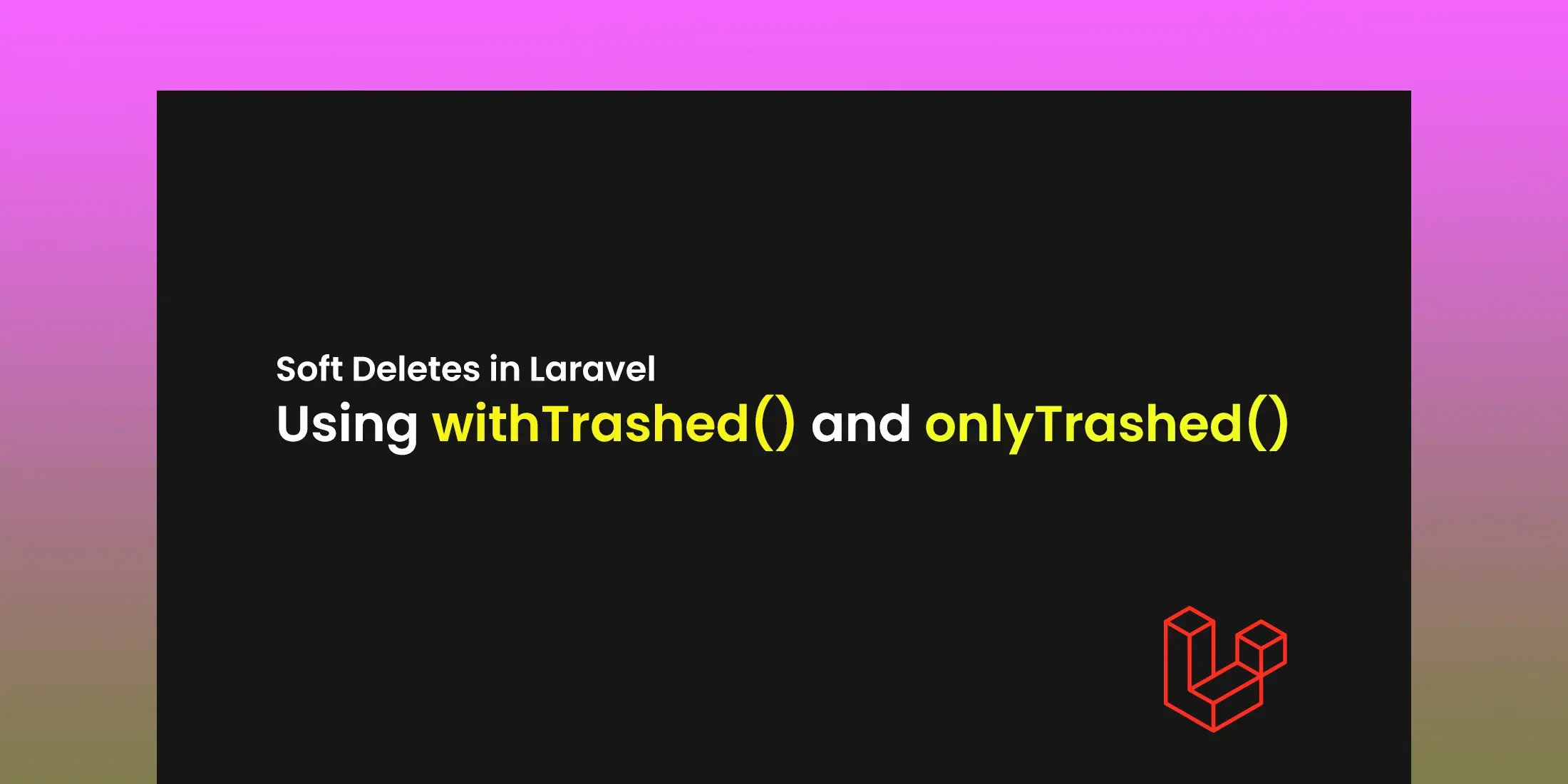
Soft Deletes in Laravel: Using withTrashed() and onlyTrashed() in Eloquent Queries
Laravel's Eloquent ORM has many features, soft deleting is one of the most useful when it comes to managing data.Soft deletes allow developers to mark records as deleted without actually removing them from the database, which can be crucial for applications that need to retain all the data including the data before and after the changes have been made. This article will delve into two methods that are essential when working with soft deletes: withTrashed() and onlyTrashed().
1. Understanding Soft Deletes in Laravel
It's important to have a clear understanding of what soft deletes are and how they work in Laravel before we discuss withTrashed() and onlyTrashed(). In a traditional delete operation, the data is permanently removed from the database but there are instances where you might want to keep the record but mark it as "deleted." This is where soft deletes come in handy.
When you use soft deletes, Laravel does not actually remove the record from the database. Instead, it sets a deleted_at timestamp on the record, indicating when it was deleted. This timestamp is managed by Laravel, and any queries made on the model will automatically exclude records with a deleted_at value unless specified otherwise.
To activate soft deletes on a model, you simply add the SoftDeletes trait to your Eloquent model. The code for add the SoftDeletes trait to your Eloquent model is given below :
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model
{
useSoftDeletes;
protected $dates = ['deleted_at'];
}
By using the above code whenever you call the delete() method on a Post model, the record will not be removed from the database but will have its deleted_at column set to the timestamp.
2. The withTrashed() Method: Retrieving All Records
By default, any query on a model with soft deletes enabled will exclude soft-deleted records. However, there are cases where you might want to include them.To retrieve both soft-deleted and non-deleted records in a query the withTrashed() method is used.
For example, an admin panel where an administrator needs to view all posts, including those that have been soft-deleted. This is where withTrashed() comes in handy.
$posts = Post::withTrashed()->get();
In the above example, the query retrieves all posts from the posts table, including those that have been soft-deleted. This method can be combined with other query builder methods to create more specific queries.
Practical Scenario: Imagine you're building a content management system (CMS) where administrators have the ability to review all posts, including those that have been soft-deleted. By using withTrashed(), you can easily create a view that lists all posts, allowing administrators to restore or permanently delete posts as needed by using the code given below:
$posts = Post::withTrashed()->where('author_id', $authorId)->get();
This query retrieves all posts by a specific author, regardless of whether they have been soft-deleted.
3. The onlyTrashed() Method: Focusing on Soft-Deleted Records
The onlyTrashed() method does the opposite of withTrashed(); it retrieves only the soft-deleted records. This method is useful in instances where you want to manage or restore soft-deleted items.
$deletedPosts = Post::onlyTrashed()->get();
In the above example, only the posts that have been soft-deleted are retrieved. This is perfect for implementing features like a "Trash" or "Recycle Bin" where users can view and restore deleted items.
Practical Scenario:Imagine a blogging platform that allows users to delete posts temporarily. These posts should be moved to a "Trash" section, from which they can be either restored or permanently deleted. By using onlyTrashed(), you can easily fetch all soft-deleted posts and display them in the Trash sectionby using the code given below:
$deletedPosts = Post::onlyTrashed()->where('author_id', $authorId)->get();
This query retrieves all posts by a specific author that have been soft-deleted, which could be displayed in a "Trash" section.
4. Restoring Soft-Deleted Records
After retrieving soft-deleted records using onlyTrashed(), you can also restore them. Laravel provides a restore() method for this purpose, which removes the deleted_at timestamp and makes the record active again.
In the above example, the soft-deleted post with the specified ID is restored, and its deleted_at timestamp is set to null.
Bulk Restore:
If you need to restore multiple records at once, you can use a bulk restore operation by using the following code:
Post::onlyTrashed()->where('author_id', $authorId)->restore();
This query restores all soft-deleted posts for a specific author. Bulk restore operations are useful when managing a large number of soft-deleted records, such as when an admin decides to restore all deleted content by a particular user.
5. Permanently Deleting Soft-Deleted Records
To permanently delete soft-deleted records from the database, you can use the forceDelete() method. This method removes the record entirely, bypassing the soft delete mechanism.
Example of Usage:
$deletedPost = Post::onlyTrashed()->find($postId);
$deletedPost->forceDelete();
In the above example, the soft-deleted post is permanently removed from the database. This action is irreversible, so it should be used with caution.
Bulk Force Delete:
Bulk force delete query permanently deletes all soft-deleted posts for a specific author by using the following code:
Post::onlyTrashed()->where('author_id', $authorId)->forceDelete();
6. Combining withTrashed() and onlyTrashed() for Advanced Queries
You can combine withTrashed() and onlyTrashed() with other query builder methods to create more complex and tailored queries. For example, if you want to retrieve all posts, including soft-deleted ones, except those belonging to a specific author, you can do so by using the following code:
$posts = Post::withTrashed()->where('author_id', '!=', $excludedAuthorId)->get();
Also if you want to retrieve only the soft-deleted posts except those from a specific authoryou can do so by using the following code:
$deletedPosts = Post::onlyTrashed()->where('author_id', '!=', $excludedAuthorId)->get();
These combinations provide you the flexibility to manage your data in a way that suits your application's needs, providing control over which records are retrieved and manipulated.
7. Practical Applications of withTrashed() and onlyTrashed()
The withTrashed() and onlyTrashed() methods have numerous practical applications in real-world scenarios:
Admin Panels: Allow administrators to view, restore, or permanently delete soft-deleted records. This is essential for maintaining data integrity and giving admins the ability to manage all content, including content that users have soft-deleted.
Trash or Recycle Bin Features: Implement a trash feature where users can view and restore their deleted items. This can provides a way to recover deleted data.
Audit Trails: Track when records were soft-deleted and by whom, and potentially allow these records to be restored. This is particularly useful in applications that require audit logs.
Summary
Laravel's withTrashed() and onlyTrashed() are tools for managing soft-deleted records in your application. They provide the ability to include or exclude soft-deleted records in your queries, allowing you to build powerful and user-friendly applications. Whether you're developing an admin panel, implementing a trash feature, or simply handling soft-deleted data, these methods offer the control you need to manage your database records effectively.
By using these methods you can create applications that are flexible and also more resistant to user errors and data loss. With withTrashed() and onlyTrashed(), you can manage your data with precision.