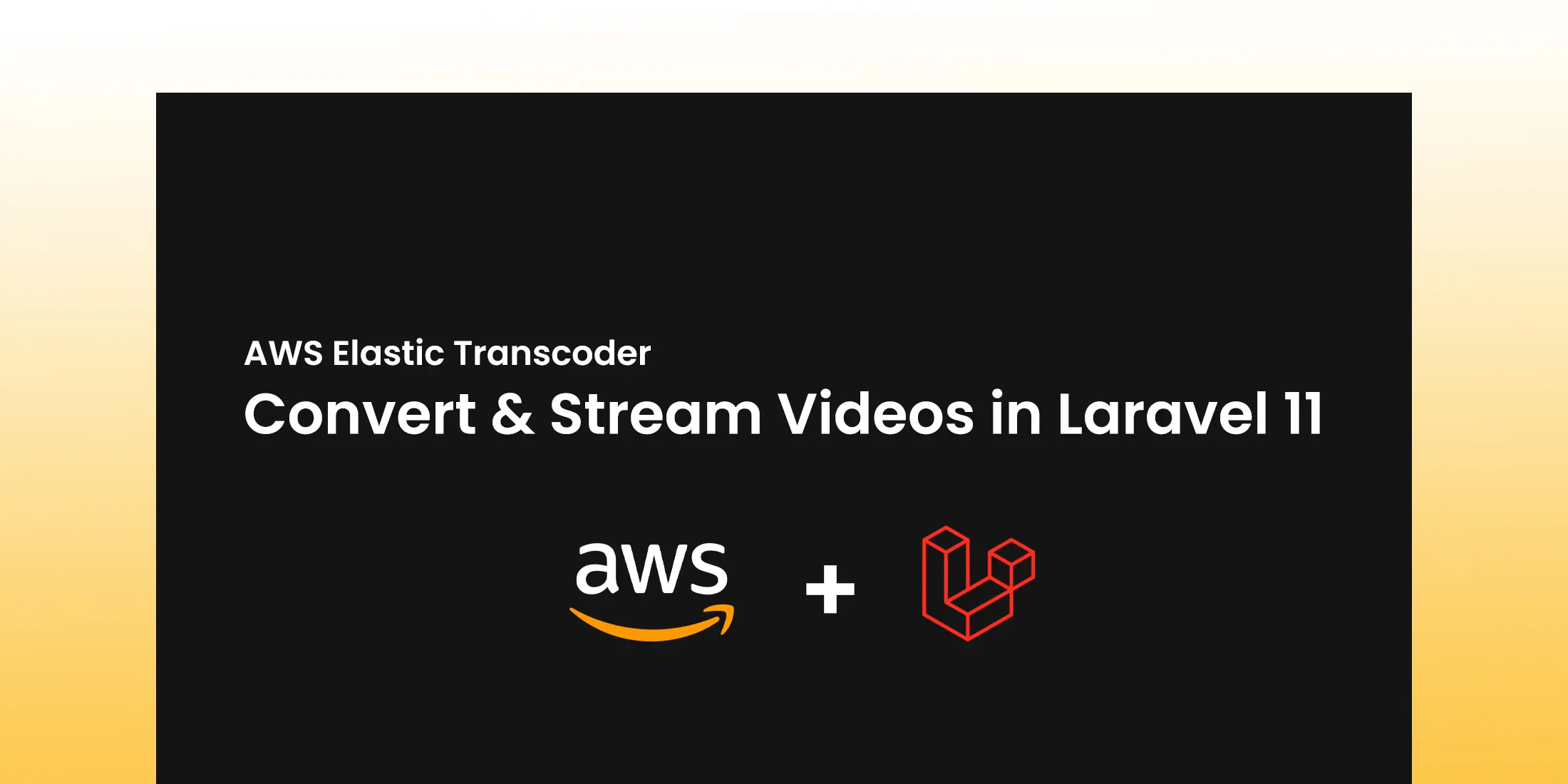
AWS Elastic Transcoder: Convert & Stream Videos in Laravel 11
To play videos online on various devices and network conditions requires converting them to be playable on multiple platforms.AWS Elastic Transcoder is a powerful tool used for transcoding media files in the cloud.In this guide, we'll explore how to integrate AWS Elastic Transcoder into a Laravel 11 project. We’re going to guide you from start to finish.
Step 1: Install the AWS SDK
Install the AWS SDK for PHP, which will provide the necessary tools to interact with AWS services like S3 and Elastic Transcoder.
php composer require aws/aws-sdk-php
Step 2: Set Up AWS Credentials
Set up your AWS credentials in your Laravel .env file. These will be used to authenticate your requests to AWS services.
AWS_ACCESS_KEY_ID=your_aws_access_key_id
AWS_SECRET_ACCESS_KEY=your_aws_secret_access_key
AWS_DEFAULT_REGION=your_aws_region
AWS_BUCKET=your_s3_bucket_name
AWS_PIPELINE_ID=your_elastic_transcoder_pipeline_id
AWS_S3_BUCKET_URL=https://your_s3_bucket_url/
Step 3: Configure S3 Disk in config/filesystems.php
To interact with S3 for uploading and managing video files, you need to configure the S3 disk in Laravel.
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
'url' => env('AWS_URL'),
'endpoint' => env('AWS_ENDPOINT'),
'use_path_style_endpoint' => env('AWS_USE_PATH_STYLE_ENDPOINT', false),
'throw' => false,
]
Step 4: Create the Video Transcoding Job
Create a job to handle video transcoding using AWS Elastic Transcoder. The following code defines a TranscodeVideoJob that will be conveyed when a video needs to be transcoded. Job Creation : First, create the job class:
php artisan make:job TranscodeVideoJob
Implementing the Job : Given below is the execution of the TranscodeVideoJob. This job handles the process of submitting a video for transcoding to AWS Elastic Transcoder and updating the video model with the outcomes.
<?php
namespace App\Jobs;
use Carbon\Carbon;
use App\Models\AWSStreamMedia;
use Illuminate\Bus\Queueable;
use Illuminate\Support\Facades\Log;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Storage;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Contracts\Queue\ShouldBeUnique;
use Aws\ElasticTranscoder\ElasticTranscoderClient;
class TranscodeVideoJob implements ShouldQueue, ShouldBeUnique
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
/**
* Create a new job instance.
*/
public $video;
public $tries = 1;
public $maxExceptions = 3;
public $timeout = 30000;
public function __construct(AWSStreamMedia $video)
{
$this->video = $video;
}
/**
* Execute the job.
*/
public function handle(): void
{
$inputKey = $this->video->video_key; //video_key contains the url of already uploaded video on AWS
$outputKeyPrefix = 'stream-transcoded-videos/vid-' . $this->video->id . '/';
$segmentDuration = '10';
$s3Client = array(
'region' => env('AWS_DEFAULT_REGION'),
'version' => 'latest',
'credentials' => [
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
],
);
$transcoderClient = new ElasticTranscoderClient($s3Client);
$hls_144_preset_id = '1713xxxxxxxxx-r2w7gf'; // 144p
$hls_240_preset_id = '1713xxxxxxxxx-u3l90z'; // 240p
$hls_360_preset_id = '1713xxxxxxxxx-xdme5a'; // 360p
$hls_480_preset_id = '1713xxxxxxxxx-p8kk15'; // 480p
$hls_720_preset_id = '1713xxxxxxxxx-8ghkr5'; // 720p
$hls_1080_preset_id = '1713xxxxxxxxx-zeaaic'; // 1080p
$hls_presets = [
'hls144' => $hls_144_preset_id,
'hls240' => $hls_240_preset_id,
'hls360' => $hls_360_preset_id,
'hls480' => $hls_480_preset_id,
'hls720' => $hls_720_preset_id,
'hls1080' => $hls_1080_preset_id,
];
$outputs = [];
foreach ($hls_presets as $prefix => $preset_id) {
array_push($outputs, [
'Key' => $prefix . '_' . $this->video->id,
'PresetId' => $preset_id,
'SegmentDuration' => $segmentDuration,
]);
};
$playlist = [
'Name' => 'hls_' . $this->video->id,
'Format' => 'HLSv3',
'OutputKeys' => array_map(function ($x) {
return $x['Key'];
}, $outputs)
];
//Create the job.
$create_job_result = $transcoderClient->createJob([
'PipelineId' => env('AWS_PIPELINE_ID'),
'Input' => ['Key' => $inputKey],
'Outputs' => $outputs,
'OutputKeyPrefix' => $outputKeyPrefix,
'Playlists' => [$playlist],
]);
$data = $create_job_result->toArray();
if ($data['@metadata']['statusCode'] === 201) {
$jobId = $data['Job']['Id'] ?? '';
$playlist = json_encode($data['Job']['Playlists']) ?? '';
$playLink = '';
if ($playlist) {
$playlistArray = json_decode($playlist, true);
if (isset($playlistArray[0]['Name'])) {
$playLink = $playlistArray[0]['Name'];
}
}
if ($playLink) {
$videoUrl = env('AWS_S3_BUCKET_URL') . 'stream-transcoded-videos/vid-' . $this->video->id . '/' . $playLink . '.m3u8';
} else {
$videoUrl = "error";
}
// After Transcoding deleting the old video
if ($inputKey && Storage::disk('s3')->exists($inputKey)) {
Storage::disk('s3')->delete($inputKey);
}
// Saving the video url in DB
$this->video->update([
'video_converted_at' => Carbon::now(),
'play_link' => $videoUrl,
]);
Log::info('Transcoding Job Completed Successfully.');
} else {
Log::error('Error creating job. Status code: ' . $data['@metadata']['statusCode'] . PHP_EOL);
}
}
}
Code Explanation
● AWS Client Setup: The task starts by setting up the AWS Elastic Transcoder client with your credentials.
● Preset IDs: It defines the preset IDs for different HLS resolutions. These presets are specific to your AWS Elastic Transcoder setup.
● Outputs: The outputs array contains the different resolutions that the video will be transcoded into.
● Playlist: The playlist array defines how the HLS playlist will be assembled.
● Job Submission: The createJob method is used to submit the transcoding job to AWS Elastic Transcoder.
● JDatabase Update: Upon successful job creation, the job ID, playlist, and video URL are stored in the database, and the original video file is deleted from S3.
Step 5: Dispatching the Job
Once job is set up the, you can perform it when it is required in your application, normally after a user uploads a video.
public function transcodeVideo(Request $request)
{
$video = AWSVedioDetail::findOrFail($request->id);
TranscodeVideoJob::dispatch($video);
}
Conclusion
This guide has shown all the steps required to setting up AWS Elastic Transcoder in Laravel 11, from installing the necessary tools to implementing and dispatching a video transcoding job. By following these steps, you'll be able to transcode videos into multiple formats and make them available for streaming across different devices and network conditions. This setup provides a scalable solution for handling video content in your Laravel application.
For further customization, you can explore additional features of AWS Elastic Transcoder.