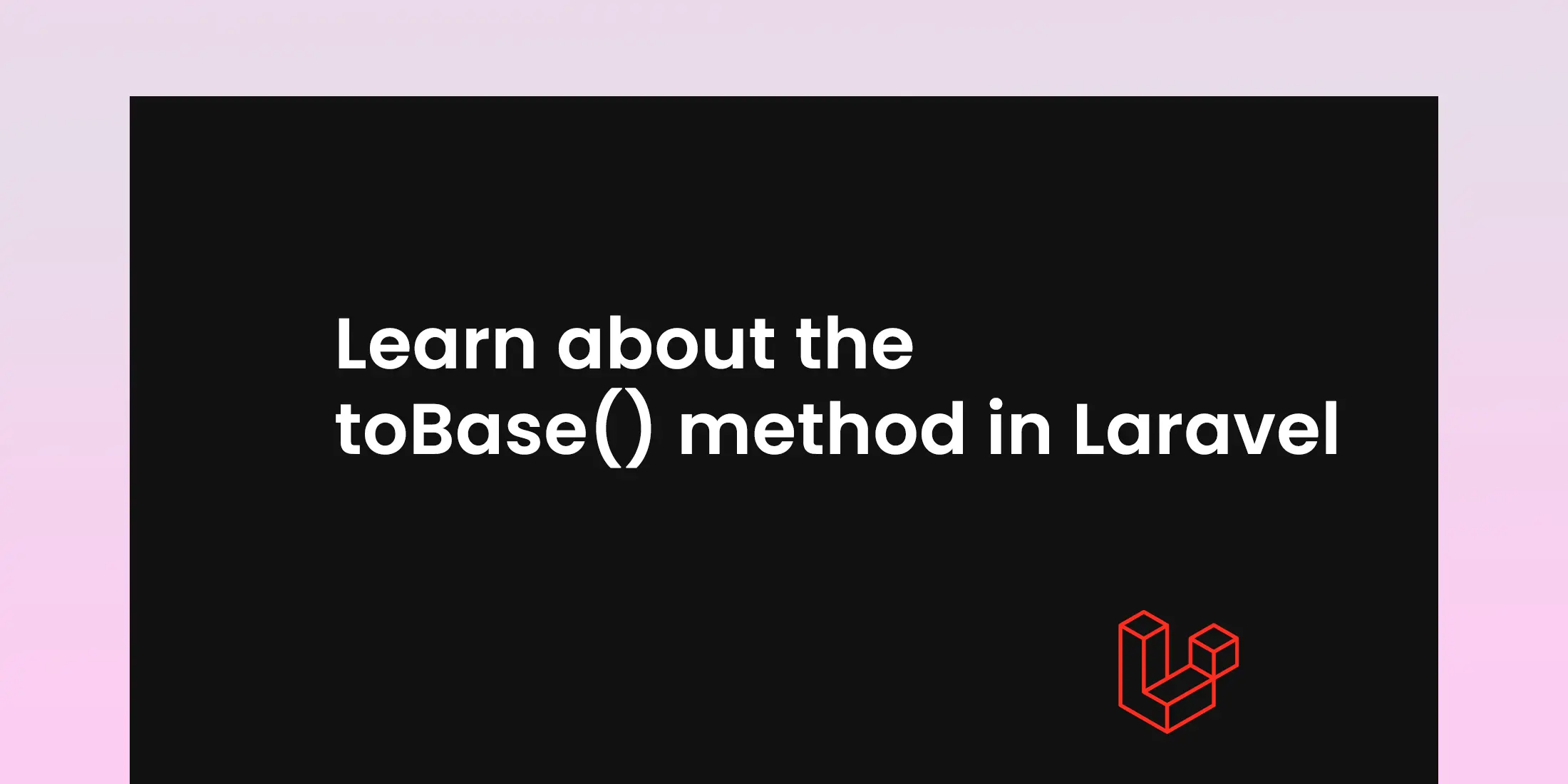
Learn about the toBase() method in Laravel - Full Guide & Examples
Laravel's ORM and Query Builder provide a convenient and powerful way to interact with your database. However, there are times when you may need to perform advanced operations that Eloquent (ORM included in Laravel is called Eloquent) can't easily handle. This is where the toBase() method comes into play, offering a way to return a query builder instance for raw SQL queries while retaining some of Laravel's convenience features.
In this tutorial we will learn what toBase() does, when and how to use it, and the benefits it offers for your Laravel projects.
What is toBase()?
The toBase() method in Laravel converts an Eloquent query builder instance into a base query builder instance. This allows you to bypass Eloquent's ORM features and directly interact with the underlying query builder, which is closer to raw SQL.
This is useful when you need to:
- Perform complex queries that Eloquent doesn't support directly.
- Optimize performance by avoiding unnecessary Eloquent model’s requirements.
- Use aggregate functions or complex joins that are troublesome with Eloquent.
Basic Usage of toBase()
Here’s a simple example to illustrate how toBase() can be used. Consider a scenario where you want to perform a raw SQL query but still take advantage of Laravel's query builder syntax.
In the above example:
- DB::table('users') starts with a typical query builder instance.
- toBase() is called to convert this into a base query builder.
- The get() method then executes the query, returning a collection of raw results.
The main difference here is that toBase() strips away Eloquent's features like eager loading and model hydration, leaving you with raw data.
Using toBase() for Performance Optimization
Sometimes, using toBase() can help optimize your queries by removing unnecessary Eloquent overhead, especially in scenarios where you're dealing with large datasets or complex queries.
In this case, the toBase() method ensures that only the raw sum is calculated, bypassing any additional processing that Eloquent would normally do.
Combining Eloquent and Raw Queries
You can also combine Eloquent features with raw queries by using toBase() in conjunction with other query builder methods. For instance, if you need to use a raw JOIN clause, but still want to work within Eloquent:
Here, toBase() allows you to execute a raw SQL join while still using the Laravel query builder for the rest of the query.
Handling Aggregate Functions with toBase()
Eloquent does not natively support some complex aggregate functions, but with toBase(), you can easily incorporate them into your queries.
In this example, toBase() allows you to directly use the max() function without Eloquent attempting to cast the result to a model.
Querying Subqueries with toBase()
Another advanced use case for toBase() is when you need to work with subqueries, which can sometimes be tricky to handle purely with Eloquent.
This example shows how toBase() can be used to manage a complex subquery and then integrate it back into a larger query.
Limitations of toBase()
While toBase() is powerful, it comes with some limitations:
- No Eloquent Model Hydration: Results are returned as raw PHP arrays or collections, not Eloquent models.
- Limited Eloquent Features: Features like relationships, attribute casting, and mutators are not available.
- Manual Handling of Results: You need to manually handle the results, including type conversions and data manipulation.
When to Use toBase()
Consider using toBase() when:
- You need to execute raw SQL queries that are too complex for Eloquent.
- You want to optimize performance by skipping Eloquent’s model hydration.
- You’re working with aggregate functions or subqueries that Eloquent doesn’t handle well.
Conclusion
Laravel's toBase() method provides a powerful way to bridge the gap between Eloquent's ease of use and the raw power of SQL. By understanding when and how to use toBase(), you can write more efficient, flexible, and complex database queries while still enjoying the benefits of Laravel's query builder.