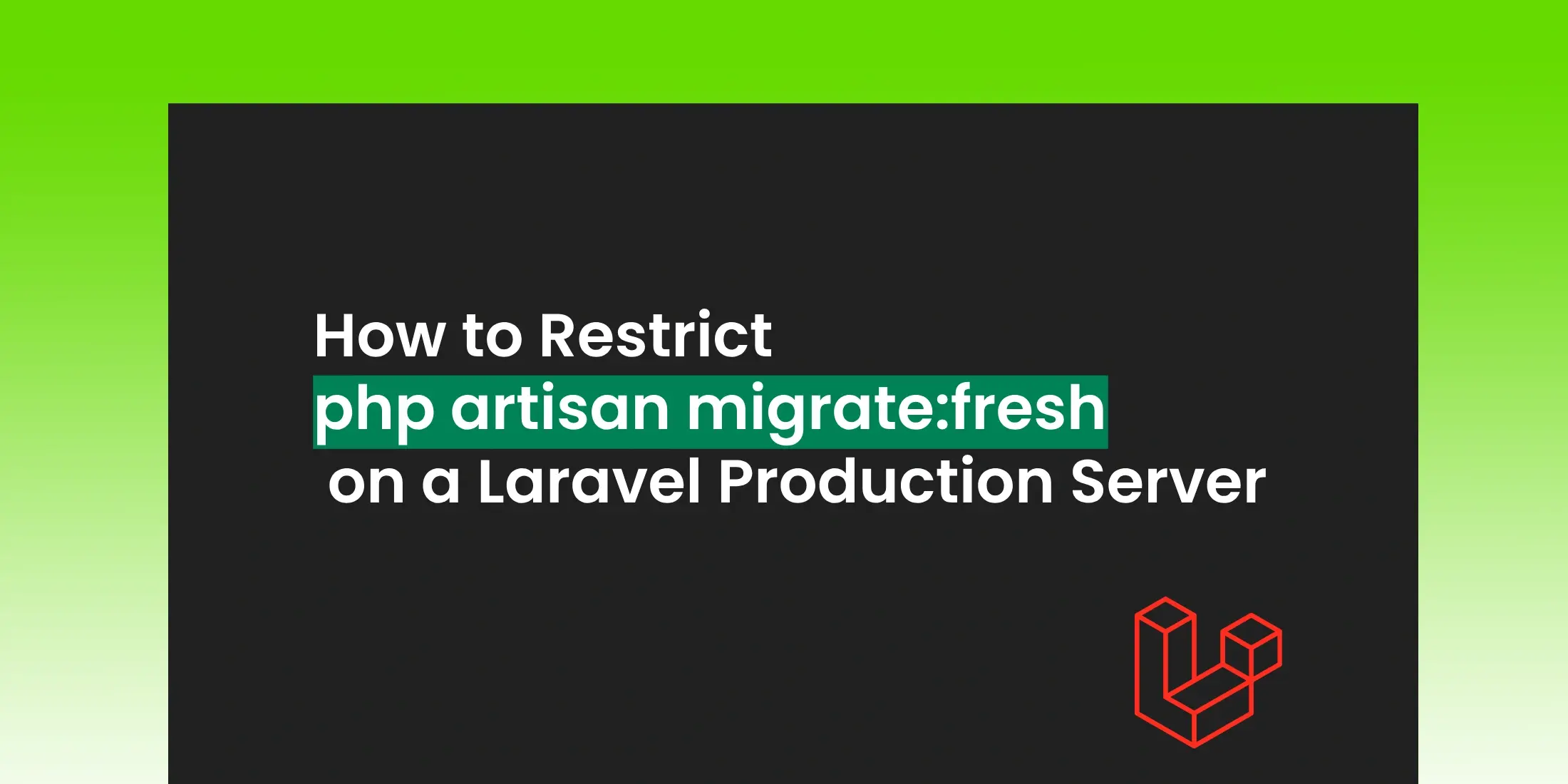
How to Restrict php artisan migrate:fresh on a Laravel Production Server
To prevent accidental data loss in your production environment, Laravel provides built-in ways to restrict destructive database commands, such as:
php artisan migrate:fresh
php artisan migrate:refresh
php artisan migrate:reset
php artisan db:wipe
Step 1: Set Environment to Production
Make sure your production .env file contains:
APP_ENV=production
Step 2: Add Restrictions in AppServiceProvider
Open app/Providers/AppServiceProvider.php and update the boot() method:
use Illuminate\Database\Console\Migrations\FreshCommand;
use Illuminate\Database\Console\Migrations\RefreshCommand;
use Illuminate\Database\Console\Migrations\ResetCommand;
use Illuminate\Database\Console\WipeCommand;
use Illuminate\Support\Facades\DB;
public function boot(): void
{
// Automatically prohibit destructive commands in production
DB::prohibitDestructiveCommands($this->app->isProduction());
// OR, individually prohibit specific commands
if ($this->app->isProduction()) {
FreshCommand::prohibit();
RefreshCommand::prohibit();
ResetCommand::prohibit();
WipeCommand::prohibit();
}
}
What This Does
DB::prohibitDestructiveCommands(true)
→ Laravel will automatically restrict commands like:
migrate:fresh
migrate:refresh
migrate:reset
db:wipe
You can also prohibit them manually (as shown) for more control or clarity.
If you try to run a restricted command in production, you’ll see:
Command prohibited in production environment.
⚠️ Important: Never run destructive commands like migrate:fresh in production without fully understanding the consequences. These commands will delete all your data.
Example for Custom Artisan Commands
If you're creating your own destructive command, you can restrict it like this:
use Illuminate\Console\Command;
use Illuminate\Console\Concerns\Prohibitable;
class SomeDestructiveCommand extends Command
{
use Prohibitable;
protected $signature = 'custom:destructive-action';
public function handle()
{
// your destructive logic here
}
}
Then in your AppServiceProvider, you can call:
SomeDestructiveCommand::prohibit($this->app->isProduction());
By following these steps, you safeguard your production database from destructive mistakes while maintaining flexibility in development environments.