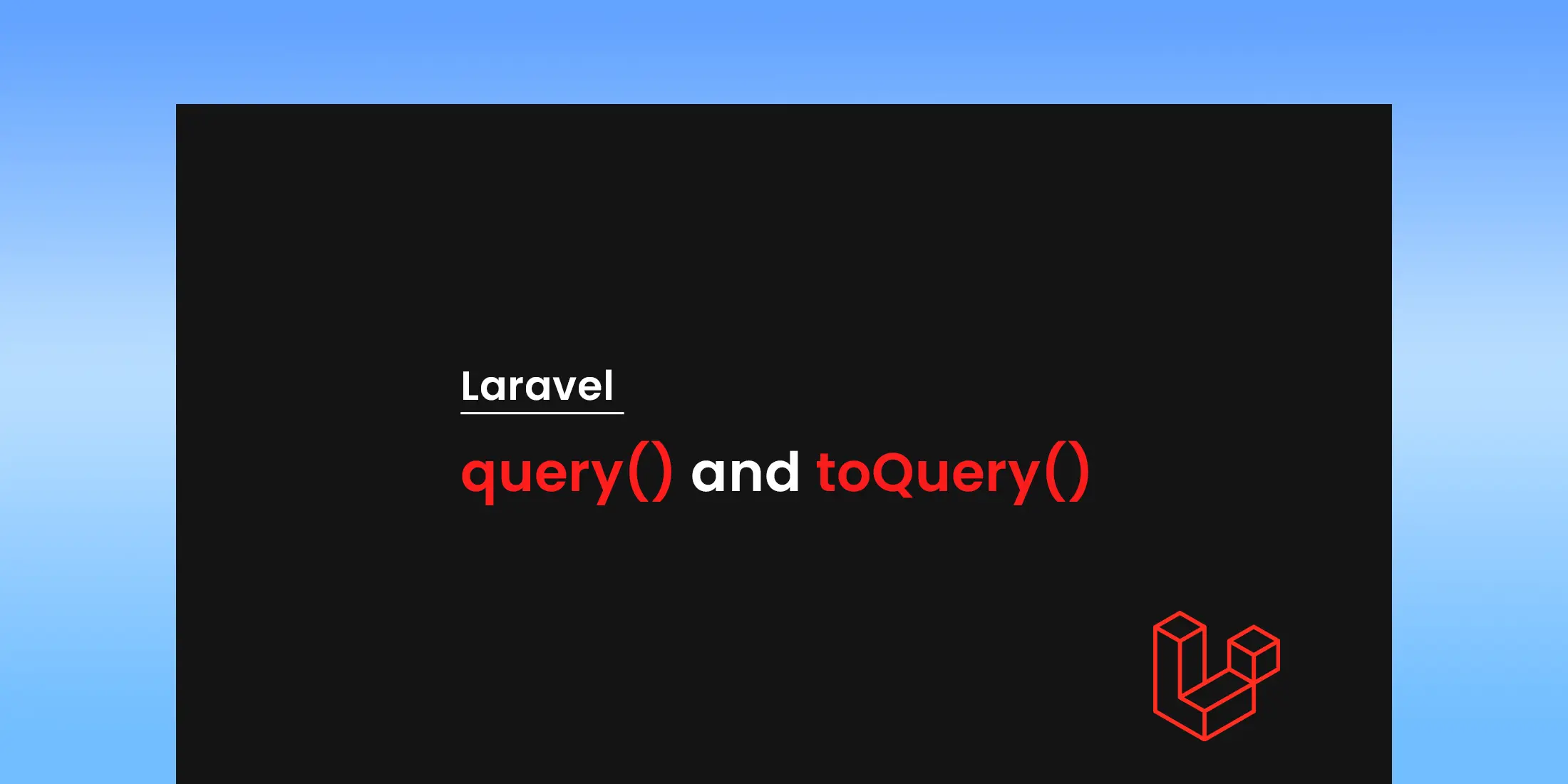
Laravel query() and toQuery() Explained in Detail with Practical Example
In Laravel, query() and toQuery() are both used to retrieve data from the database, but they are used in different contexts., choosing between query() and toQuery() depends on what you're working with and your specific needs. Both methods have different uses one is not better than the other. We are going to walk you through the differences between these methods, their use cases, and provide examples to clarify their usage.
What is query() in Laravel?
The query() method in Laravel is used to launch a query on an Eloquent model or a database table. It allows developers to write complex queries that might go beyond typical Eloquent ORM methods. This method provides access to Laravel's query builder, enabling more flexibility in retrieving data.
Features of query():
- Can be used on models or tables directly.
- Used for more complex database interactions.
- Provides a query builder for easily crafting queries.
- Does not return Eloquent collections but rather raw results or query builders.
Example 1: Retrieving all users with raw query
$users = DB::table('users')->query()->where('active', 1)->get();
The above example uses query() to perform a join between the posts and users tables and retrieves the post titles and corresponding user names.
Example 2: Using Eloquent to make a custom query
$posts = Post::query()->where('status', 'published')->orderBy('created_at', 'desc')->get();
Here, Post::query() returns an instance of the query builder for the posts model, allowing you to chain custom SQL-like queries and retrieve results.
Example 3: Joining tables with query()
$posts = DB::table('posts')->query()
->join('users', 'posts.user_id', '=', 'users.id')
->select('posts.title', 'users.name')
->get();
The above example uses query() to perform a join between the posts and users tables and retrieves the post titles and corresponding user names.
What is toQuery() in Laravel?
While query() is used for building queries on models and tables, toQuery() is specifically designed to handle Eloquent relationships. It converts an Eloquent relationship (like has Many, belongs To, etc.) into a query builder, enabling developers to add additional conditions or manipulate the relationship data without immediately loading it.
Features of toQuery():
- Typically used on relationships (e.g., has Many, belongs To).
- Transforms the relationship into a query builder to apply further conditions.
- Helps you perform queries on related models before executing the final SQL.
Example 1: Accessing a related model’s query builder
$user = User::find(1);
$posts = $user->posts()->toQuery()->where('status', 'published')->get();
In the above example, toQuery() converts the posts relationship (which is a hasMany relationship on User) into a query builder. You can then apply conditions like filtering published posts.
Example 2: Querying a relationship without eager loading
$comments = Post::find(1)->comments()->toQuery()->where('approved', true)->get();
In the above example, toQuery() is used on the comments relationship of a Post model to filter only approved comments. This avoids eager loading the entire relationship data.
Example 3: Counting related models with toQuery()
$user = User::find(1);
$publishedPostsCount = $user->posts()->toQuery()->where('status', 'published')->count();
In the above example case, toQuery() allows you to count the number of related posts where the status is 'published', instead of loading all posts into memory.
Difference between query() and toQuery() in Laravel
Method: query()
Description: Used for building complex SQL queries on Eloquent models or tables.
Usage: Available directly on models or database tables.
Return Type: Query builder object or raw SQL results.
Method: toQuery()
Description: Used for querying relationships without eager loading the data.
Usage: Available on Eloquent relationships.
Return Type: Query builder object for relationships.
Understanding the difference between query() and toQuery() can help you write more efficient and optimized queries in Laravel. While query() is perfect for complex, raw queries on models or tables, toQuery()is best used when you need to manipulate relationships before retrieving data.
query() is better for building fresh queries on the base model.toQuery() is more efficient when you're dealing with Eloquent relationships and want to avoid loading related models into memory.