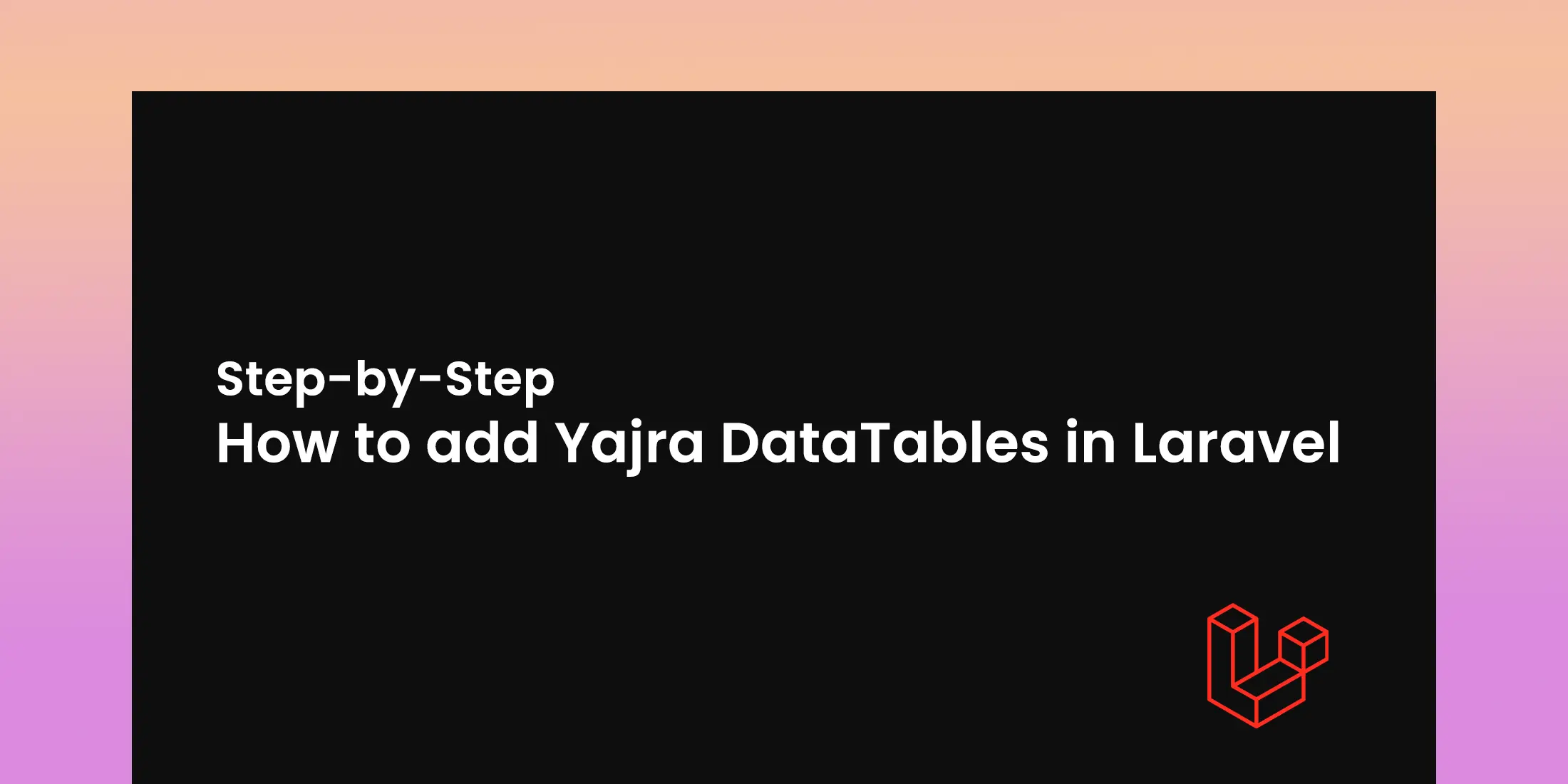
how to add Yajra DataTables in Laravel Step-by-Step Guide
In this simple step-by-step guide, we will show you how to add Yajra DataTables to Laravel application. This powerful tool helps you manage large amounts of data easily and efficiently.
You’ll learn how to start with a new Laravel project, connect your database, and use the built-in users table. We’ll walk you through the process of installing Yajra DataTables and setting up everything you need for smooth server-side data handling.
By the end of this tutorial, you’ll have a working DataTable in your Laravel app that makes it easy to view and manage user information. Whether you're building a small project or a larger application, Yajra DataTables will make your data management much easier.
Installation
composer create-project laravel/laravel yajra-demo
Navigate to your yajra-demo folder by using
cd yajra-demo
Database Setup
Make sure to connect your db by going into your .env file
We will use the default users table.
Yajra Datatables Integration and Configuration
This command give you the latest version
composer require yajra/laravel-datatables-oracle:"^10.3.1"
This step is optional if you are using Laravel 5.5+
Open the file config/app.php'providers' => ServiceProvider::defaultProviders()->merge([
Yajra\DataTables\DataTablesServiceProvider::class,
])->toArray(),
Publish configuration and assets.
php artisan vendor:publish --tag=datatables
Configure Scripts and CSS
resources\js\bootstrap.jsimport 'bootstrap';
import 'laravel-datatables-vite';
resources/sass/app.scss
// Fonts
@import url('https://fonts.bunny.net/css?family=Nunito');
// Variables
@import 'variables';
// Bootstrap
@import 'bootstrap/scss/bootstrap';
// DataTables
@import 'bootstrap-icons/font/bootstrap-icons.css';
@import "datatables.net-bs5/css/dataTables.bootstrap5.min.css";
@import "datatables.net-buttons-bs5/css/buttons.bootstrap5.min.css";
@import 'datatables.net-select-bs5/css/select.bootstrap5.css';
Authentication
Now install the Laravel ui for authentication
php artisan ui bootstrap
php artisan ui bootstrap --auth
npm install
Once the dependencies have been installed using npm install, now we need to compile SASS files to plain CSS using Vite.
npm run dev
Create User Datatable
php artisan datatables:make Users
Navigate to your app\DataTables\UsersDataTable.php
namespace App\DataTables;
use App\Models\User;
use Illuminate\Database\Eloquent\Builder as QueryBuilder;
use Yajra\DataTables\EloquentDataTable;
use Yajra\DataTables\Html\Builder as HtmlBuilder;
use Yajra\DataTables\Html\Button;
use Yajra\DataTables\Html\Column;
use Yajra\DataTables\Services\DataTable;
class UsersDataTable extends DataTable
{
/**
* Build the DataTable class.
*
* @param QueryBuilder $query Results from query() method.
*/
public function dataTable(QueryBuilder $query): EloquentDataTable
{
return (new EloquentDataTable($query))
->addColumn('action', 'users.action')
->setRowId('id');
}
/**
* Get the query source of dataTable.
*/
public function query(User $model): QueryBuilder
{
return $model->newQuery();
}
/**
* Optional method if you want to use the html builder.
*/
public function html(): HtmlBuilder
{
return $this->builder()
->setTableId('users-table')
->columns($this->getColumns())
->minifiedAjax()
//->dom('Bfrtip')
->orderBy(1)
->selectStyleSingle()
->buttons([
Button::make('excel'),
Button::make('csv'),
Button::make('pdf'),
Button::make('print'),
Button::make('reset'),
Button::make('reload')
]);
}
/**
* Get the dataTable columns definition.
*/
public function getColumns(): array
{
return [
Column::make('id'),
Column::make('name'),
Column::make('email'),
// Column::computed('action')
// ->exportable(false)
// ->printable(false)
// ->width(60)
// ->addClass('text-center'),
];
}
/**
* Get the filename for export.
*/
protected function filename(): string
{
return 'Users_' . date('YmdHis');
}
}
Note
* If you want to modify some column you use **dataTable()** function. * In **getColumns()**, you need to specify which columns you wanted to show. * If you want to customize your query , you will use **query()** function. * **html()** This is optional if you want to see these html method.Setup a Users Controller, View & Route
App\Http\Controllers\HomeController
// public function index()
// {
// return view('home');
// }
public function index(UsersDataTable $dataTable)
{
return $dataTable->render('home');
}
resources\views\home.blade.php
<extends>('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">{{ 'Users' }}</div>
<div class="card-body">
{{ $dataTable->table() }}
</div>
</div>
</div>
</div>
</div>
@endsection
@push('scripts')
{{ $dataTable->scripts(attributes: ["type" => "module"]) }}
@endpush
yajra is using Jquery , so need to add jQuery Link also.
resources\views\layouts\app.blade.php
{{-- Jquery --}}
<script src="https://code.jquery.com/jquery-3.7.0.min.js"></script>
@stack('scripts')
{{ $scripts ?? '' }}
Output
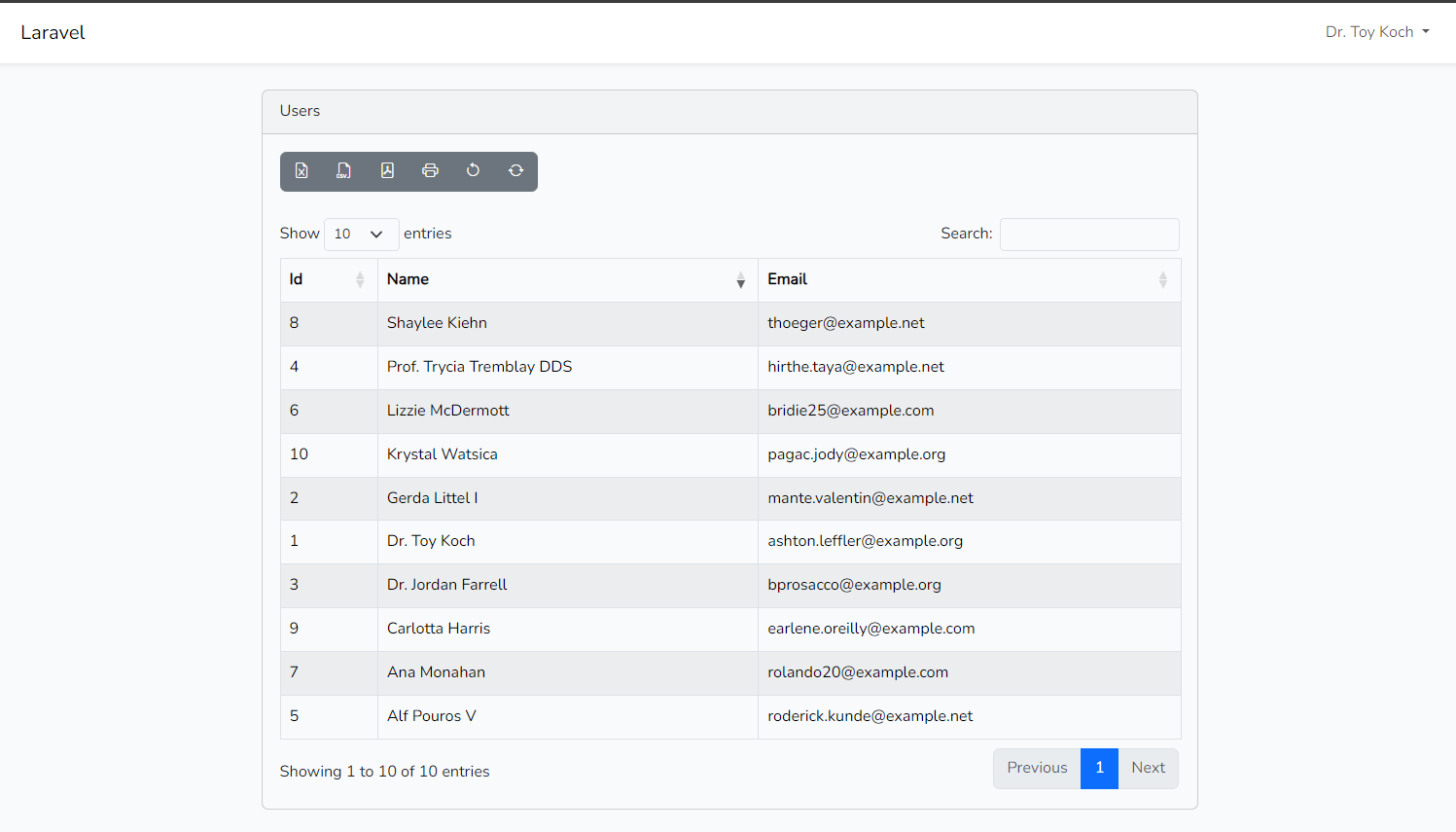
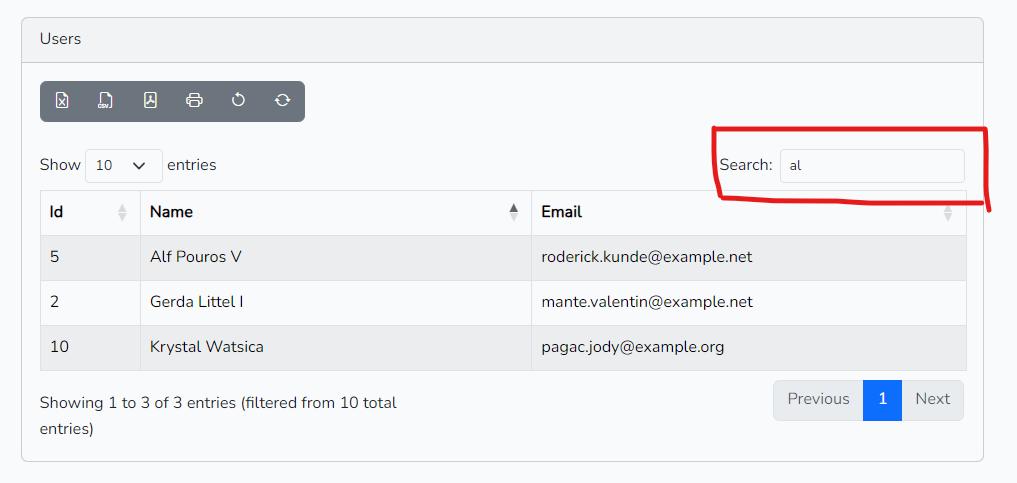