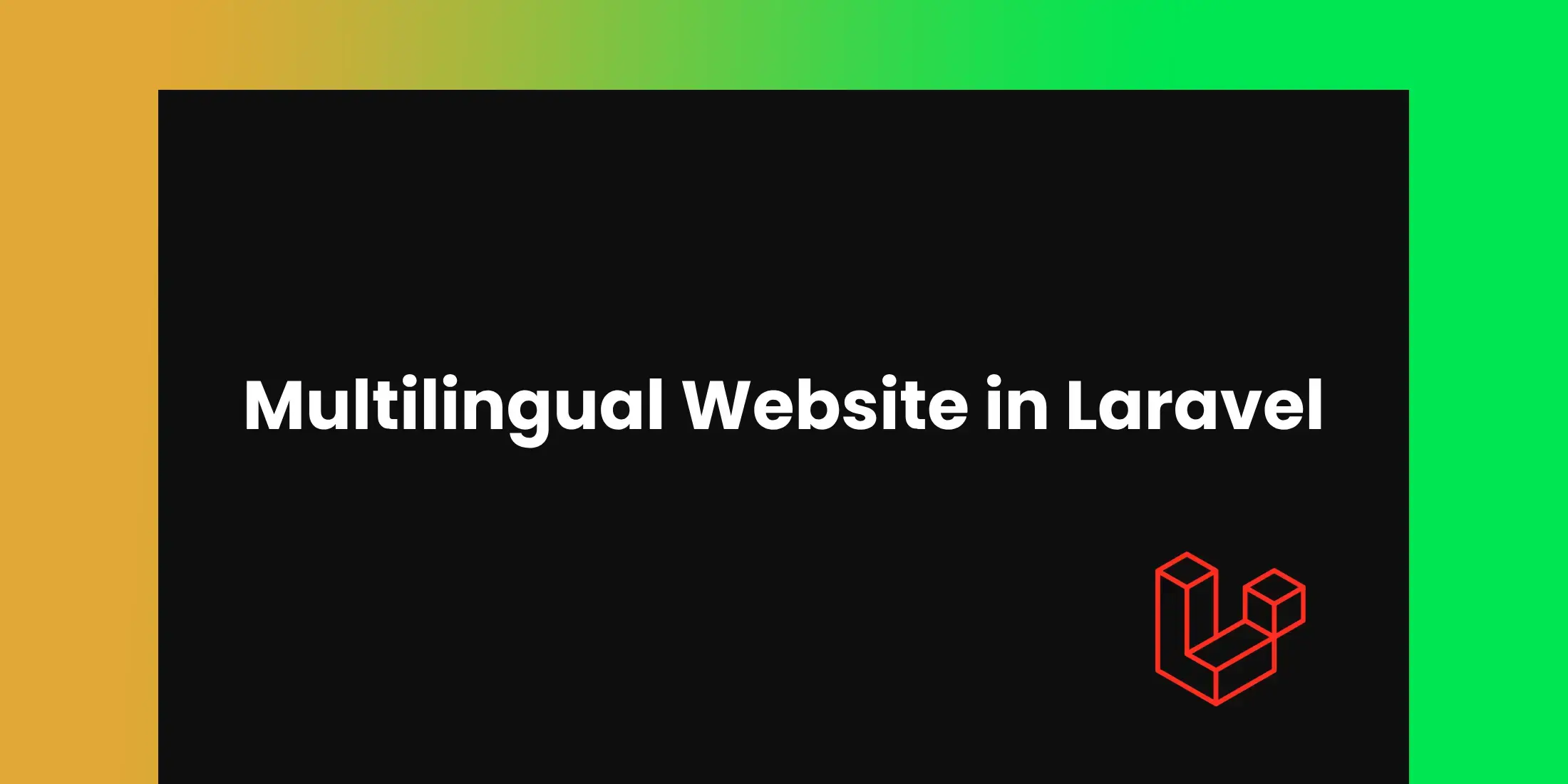
Laravel Localization: How to create a Multilingual Website in Laravel
Building a multilingual website in Laravel is super easy. Laravel with its cool features make it easy to create a website that supports multiple language.In this article we will create a multilingual website from setting up language files to adding language switchers and handling translations for dynamic content like database entries.
A multilingual website helps you reach a global audience, improves user experience, and makes your site accessible to more people.
Step 1: Setting Up Language Files
Laravel uses language files stored in the resources/lang directory. Each language that your website will support has its own subdirectory, where you'll define translation strings.
1.1 Create Language Folders
Start by creating subdirectories for each language you want to support. In this example, we'll create English and French translations:
resources/lang/en resources/lang/fr 1.2 Add Translation Files
Within each language directory, create files to store your translations. These files can be named based on sections of your website, like messages.php, auth.php, etc.
Example messages.php
for English:
return [
'welcome' => 'Welcome to our website',
'contact' => 'Contact Us',
];
Example messages.php
for French:
return [
'welcome' => 'Bienvenue sur notre site Web',
'contact' => 'Contactez-nous',
];
Laravel automatically handles this structure, and you can add as many translation strings as needed for different parts of your website.
Step 2: Accessing Translations in Views
Once you’ve set up your language files, you can access these translations in your views using Laravel’s __()
helper function or @lang()
. This allows Laravel to dynamically fetch the correct translation string based on the currently selected locale.
Here’s how you can use it in a Blade view:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<h1>{{ __('messages.welcome') }}</h1>
<a href="#">{{ __('messages.contact') }}</a>
<br><br><br>
{{-- Switch Language --}}
<a href="{{ route('lang.switch', 'en') }}">English</a>
<a href="{{ route('lang.switch', 'fr') }}">Français</a>
</body>
</html>
In this example, the __('messages.welcome')
function will automatically retrieve the appropriate language string from your messages.php file.
Step 3: Setting the Default Locale
Laravel allows you to set a default locale in the config/app.php
file. This is the language Laravel will use when no specific locale is set by the user.
3.1 Modify the Configuration
Open config/app.php and locate the following lines:
'locale' => env('APP_LOCALE', 'en'),
'fallback_locale' => env('APP_FALLBACK_LOCALE', 'en'),
'faker_locale' => env('APP_FAKER_LOCALE', 'en_US'),
Step 4: Creating Language Switching Routes
To make your website truly multilingual, you’ll need to allow users to switch between languages. This requires setting up routes and a controller to handle language changes.
4.1 Define the Route
In routes/web.php, define a route for changing the language:
Route::get('lang/{locale}', [LanguageController::class, 'switchLang'])->name('lang.switch');
Here, lang/{locale} is the dynamic part of the URL that will accept language codes like en or fr.
4.2 Create Language Controller
Next, create a controller to manage the language switch:
php artisan make:controller LanguageController
In the generated LanguageController.php file, add the following code to switch the locale:
namespace App\Http\Controllers;
use Illuminate\Support\Facades\App;
use Illuminate\Support\Facades\Session;
class LanguageController extends Controller
{
public function switchLang($locale)
{
if (in_array($locale, ['en', 'fr'])) {
App::setLocale($locale);
Session::put('locale', $locale);
}
return redirect()->back();
}
}
This controller checks if the selected language is valid, switches the application locale, and stores it in the session.
Step 5: Storing Locale in Session Using Middleware
To ensure the user’s selected language persists across multiple pages, you’ll need middleware to store the locale in the session. Middleware allows you to execute certain logic before a request is handled by the application.
5.1 Create Middleware
Run the following Artisan command to create a middleware:
php artisan make:middleware SetLocale
5.2 Update Middleware Logic
In app/Http/Middleware/SetLocale.php, modify the handle function to check the session for the locale:
public function handle(Request $request, Closure $next): Response
{
if (Session::has('locale')) {
App::setLocale(Session::get('locale'));
}
return $next($request);
}
5.3 Register Middleware
Finally, register the middleware in bootstrap/app.php so it runs on every request:
->withMiddleware(function (Middleware $middleware) {
$middleware->web(append: [
SetLocale::class,
]);
})
This middleware ensures that every request uses the locale stored in the session.
Step 6: Creating a Language Switcher in Views
Now that you have a way to switch languages, you’ll need to add a language switcher in your website’s UI to let users change languages.
In a Blade template like header.blade.php
, add links for switching between languages:
English
Français
These links will trigger the switchLang method in your LanguageController and switch the application’s language.
Step 7: Testing Your Localization Setup
Once you have everything set up, it’s important to thoroughly test your localization system to ensure it works as expected.
7.1 Testing Language Switching
Visit different pages of your site and click the language switcher links to ensure the content updates to the correct language.
7.2 Check Session Storage
You can also debug your session to make sure that the locale is being stored properly. Use @dd(Session::get('locale')) in a view or controller to output the current locale.
7.3 Testing Fallback Locale
Remove a translation string from one of your language files and ensure Laravel falls back to the default language when the string is missing.
Step 8: Optional: Translating Routes
In some cases, you might want to translate the URLs themselves, so that /contact becomes /contactez-nous in French. Laravel doesn’t natively support this out of the box, but you can either create separate routes for each language or use a package like mcamara/laravel-localization.
Step 9: Translating Dynamic Content (Database)
If your website has dynamic content stored in the database, like blog posts or product descriptions, you will need to handle translations at the database level.
9.1 Approach 1: Separate Fields for Each Language
A simple approach is to store translations in separate columns, like title_en and title_fr. When retrieving data, you can show the appropriate column based on the locale.
9.2 Approach 2: Using a Translation Package
Alternatively, you can use a package like [spatie/laravel-translatable] (https://spatie.be/docs/laravel-translatable/v6/introduction), which allows you to store translations in a single column as a JSON object and easily retrieve the correct language.
Summary
Building a multilingual website in Laravel is a great way to reach more people and make your site easier to use. This step-by-step guide will help you set up language files, add a language switcher, save the chosen language in sessions, and translate content from your database. Whether you're creating a small site or a big one, Laravel’s tools for localization make everything simple and flexible.