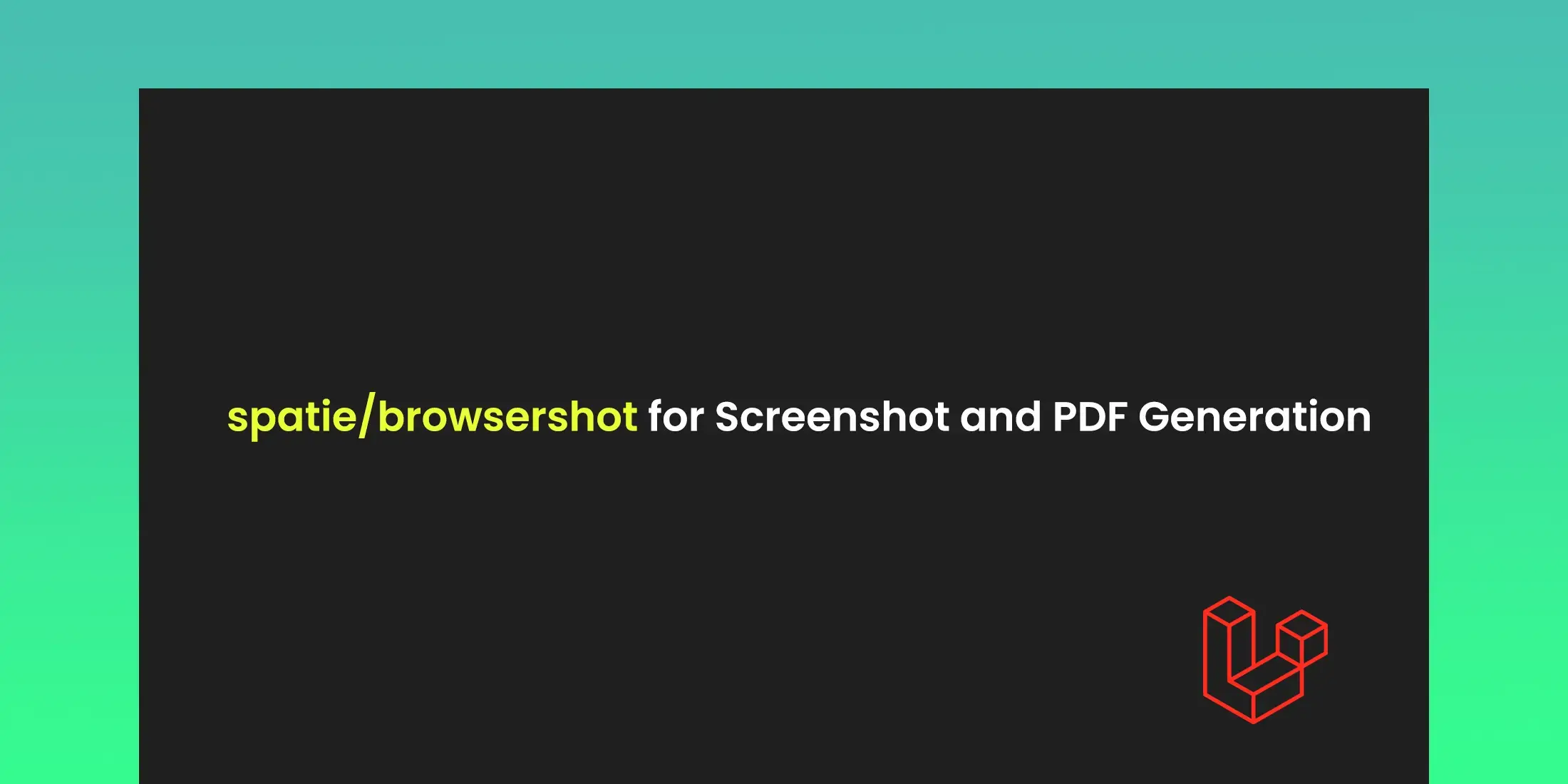
Using spatie/browsershot in Laravel for Screenshot and PDF Generation
To capture screenshots and generate PDFs from web pages in Laravel applications the spatie/browsershot package is used.It an excellent tool for generating reports, capturing visual content, or even automating testing by using leverages the headless Chrome browser to render pages and capture them as images or PDFs.
We will walk you through the process of combining integrating spatie/browsershot into your Laravel application, including installation, basic usage, and advanced features.
1. Installing the Package
To use spatie/browsershot, you need to install it via Composer. Open your terminal and run:
composer require spatie/browsershot
This command will add the package to your Laravel project.spatie/browsershot requires Node.js and the Chrome browser to be installed on your system because it uses Puppeteer to interact with a headless Chrome instance.
After installing, you need to set up the basic configuration. The default settings are usually sufficient for most use cases, you can also customize them as you require.
2. Capturing Screenshots
Capturing screenshots of web pages is a common use for spatie/browsershot. You can do it by using the following commands:
<?php
use Spatie\Browsershot\Browsershot;
class ScreenshotController extends Controller
{
public function capture()
{
$filePath = storage_path('app/screenshots/page.png');
Browsershot::url('https://www.example.com')
->setOption('width', 1200)
->setOption('height', 800)
->save($filePath);
return response()->download($filePath);
}
}
In this example, a screenshot of the specified URL is captured and saved to the storage/app/screenshots directory. The setOption methods configure the viewport size of the screenshot.
3. Generating PDFs
Generating PDFs from web pages is another feature of spatie/browsershotYou can do it by using the following commands:
<?php
use Spatie\Browsershot\Browsershot;
class PdfController extends Controller
{
public function generate()
{
$pdfPath = storage_path('app/pdfs/document.pdf');
Browsershot::url('https://www.example.com')
->setOption('printBackground', true)
->pdf($pdfPath);
return response()->download($pdfPath);
}
}
In the above example, a PDF is generated from the specified URL and saved to the storage/app/pdfs directory. The setOption('printBackground', true) method ensures that background graphics are included in the PDF.
4. Advanced Options
spatie/browsershot provides various options to customize how screenshots and PDFs are generated. Here are some advanced options you might find useful:
Viewport Size: Set the viewport size using setOption('width', 1200) and setOption('height', 800).
Full Page Screenshots: Capture the entire page, including off-screen content, with setOption('fullPage', true).
PDF Options: Customize PDF output with options like setOption('format', 'A4') to set the paper size.
use Spatie\Browsershot\Browsershot;
class AdvancedController extends Controller
{
public function captureAndGenerate()
{
// Screenshot with full page capture and viewport size
$screenshotPath = storage_path('app/screenshots/fullpage.png');
Browsershot::url('https://www.example.com')
->setOption('width', 1200)
->setOption('height', 800)
->setOption('fullPage', true)
->save($screenshotPath);
// PDF with custom options
$pdfPath = storage_path('app/pdfs/advanced_document.pdf');
Browsershot::url('https://www.example.com')
->setOption('printBackground', true)
->setOption('format', 'A4')
->pdf($pdfPath);
return response()->json([
'screenshot' => $screenshotPath,
'pdf' => $pdfPath
]);
}
}
5. Use Cases
spatie/browsershot is useful in various scenarios:
● Report Generation: Automatically generate reports and export them as PDFs for documentation or analysis.
● Web Monitoring: Capture screenshots of web pages to monitor changes or for visual documentation.
● Automated Testing: Use screenshots to capture the state of web pages during automated tests to validate UI changes.
6. Performance Considerations
Running headless Chrome instances can be resource draining. Here are some tips to manage performance:
Optimize Page Content: Minimize the content that needs to be rendered to reduce processing time.
Monitor Resource Usage: Keep an eye on CPU and memory usage, especially if generating multiple screenshots or PDFs in a short period.