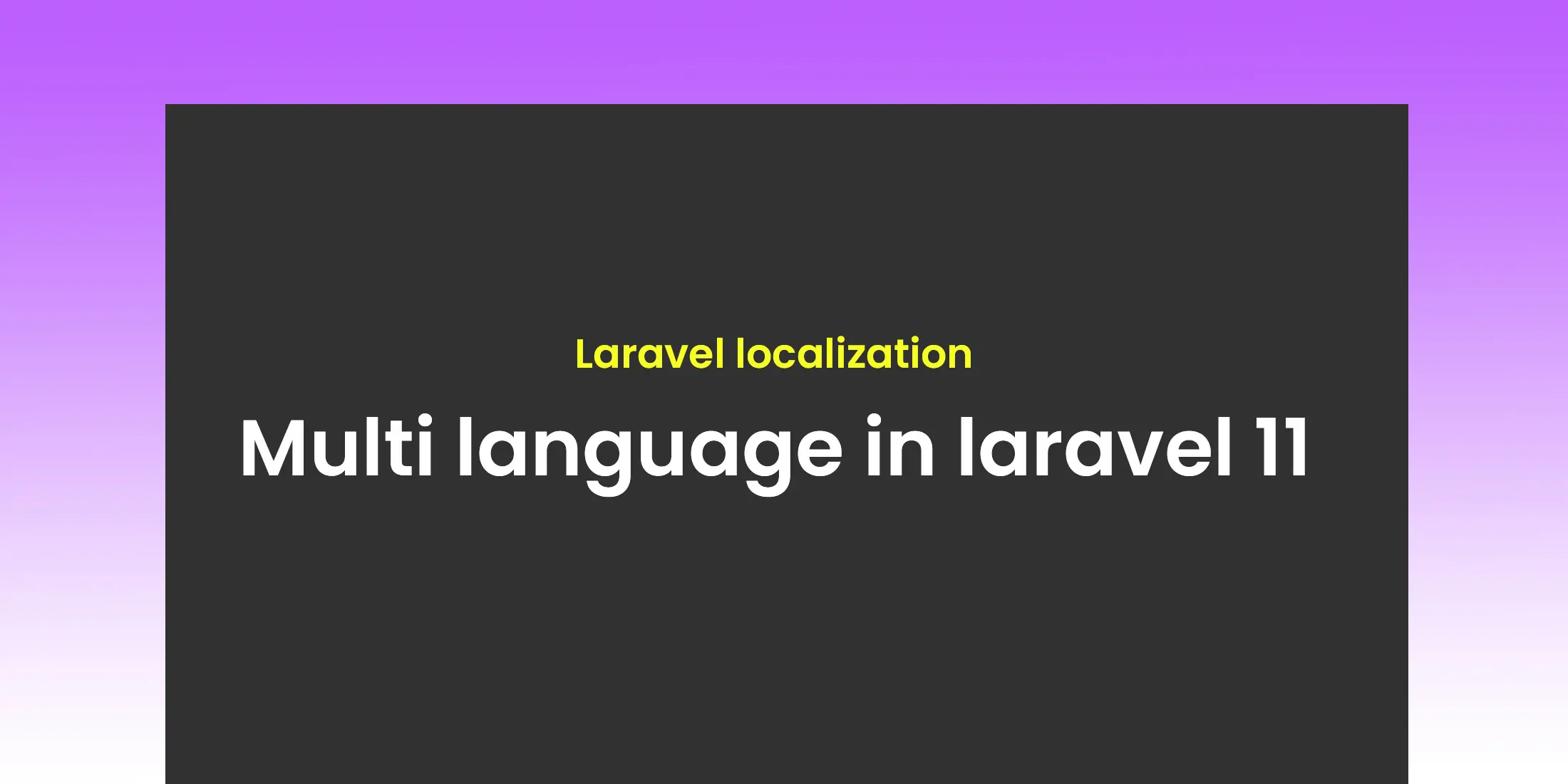
Multi language in laravel 11
In this article we will discuss localization in laravel.Multi Language is needed in almost every project here we will discuss all the basics that you need to know how we implement multi language in laravel 9,10 and 11.We will switch language based on user request and we will store that in session and then by using that session value in middleware we detect the language in laravel 8, laravel 9 laravel10 and laravel 11.
Create Language Files
Create a directory named lang
and inside that lang folder create json files inwhich you want to localize.
// lang/en.json
{
"welcome": "welcome",
"Elegant Laravel": "Elegant Laravel"
}
// lang/fr.json
{
"welcome": "bienvenu",
"Elegant Laravel": "Laravel élégant"
}
// lang/it.json
{
"welcome": "benvenuto",
"Elegant Laravel": "Elegante Laravel"
}
Our Views
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Elegant Laravel | Website</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH" crossorigin="anonymous">
</head>
<body class="d-flex h-100 text-center">
<div class="cover-container d-flex w-100 h-100 p-3 mx-auto flex-column">
<header class="mb-auto container">
<div>
<h3 class="float-md-start mb-0"><img src="" alt="" srcset=""></h3>
<nav class="nav nav-masthead justify-content-center float-md-end">
<form id="langform" action="{{ route('user.lang') }}" method="get" class="d-flex align-items-center">
<select class="form-select" name="lang" id="lang" onchange="this.form.submit()">
<option disabled>Language</option>
<option value="en" @if (Session::get('locale', 'en') == 'en') selected @endif> English</option>
<option value="fr" @if (session('locale') == 'fr') selected @endif> French</option>
<option value="it" @if (session('locale') == 'it') selected @endif> Italian</option>
</select>
</form>
</nav>
</div>
</header>
<main class="px-3">
<h1>{{ __('welcome') }}</h1>
<h1>{{ __('Elegant Laravel') }}</h1>
</main>
</div>
</body>
</html>
Route
Our route to change language in laravel.
Route::get('/lang',[LanguageController::class , 'change'])->name('user.lang');
Bussiness Logic Controller
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Session;
class LanguageController extends Controller
{
public function change(Request $request)
{
$lang = $request->input('lang');
if (!in_array($lang, ['en', 'it', 'fr'])) {
abort(400);
}
Session::put('locale', $lang);
return redirect()->back();
}
}
Set Up Middleware for Locale Switching
Now we will create a middleware that sets the locale based on user preference or request.
public function handle(Request $request, Closure $next): Response
{
if ($request->session()->has('locale')) {
App::setLocale($request->session()->get('locale', 'en'));
}
return $next($request);
}
Register the middleware in bootstrap/app.php :
<?php
use App\Http\Middleware\SetLocale;
use Illuminate\Foundation\Application;
use Illuminate\Foundation\Configuration\Exceptions;
use Illuminate\Foundation\Configuration\Middleware;
return Application::configure(basePath: dirname(_DIR_))
->withRouting(
web: _DIR_ . '/../routes/web.php',
commands: _DIR_ . '/../routes/console.php',
health: '/up',
)
->withMiddleware(function (Middleware $middleware) {
$middleware->web(append: [
SetLocale::class,
]);
})
->withExceptions(function (Exceptions $exceptions) {
//
})->create();