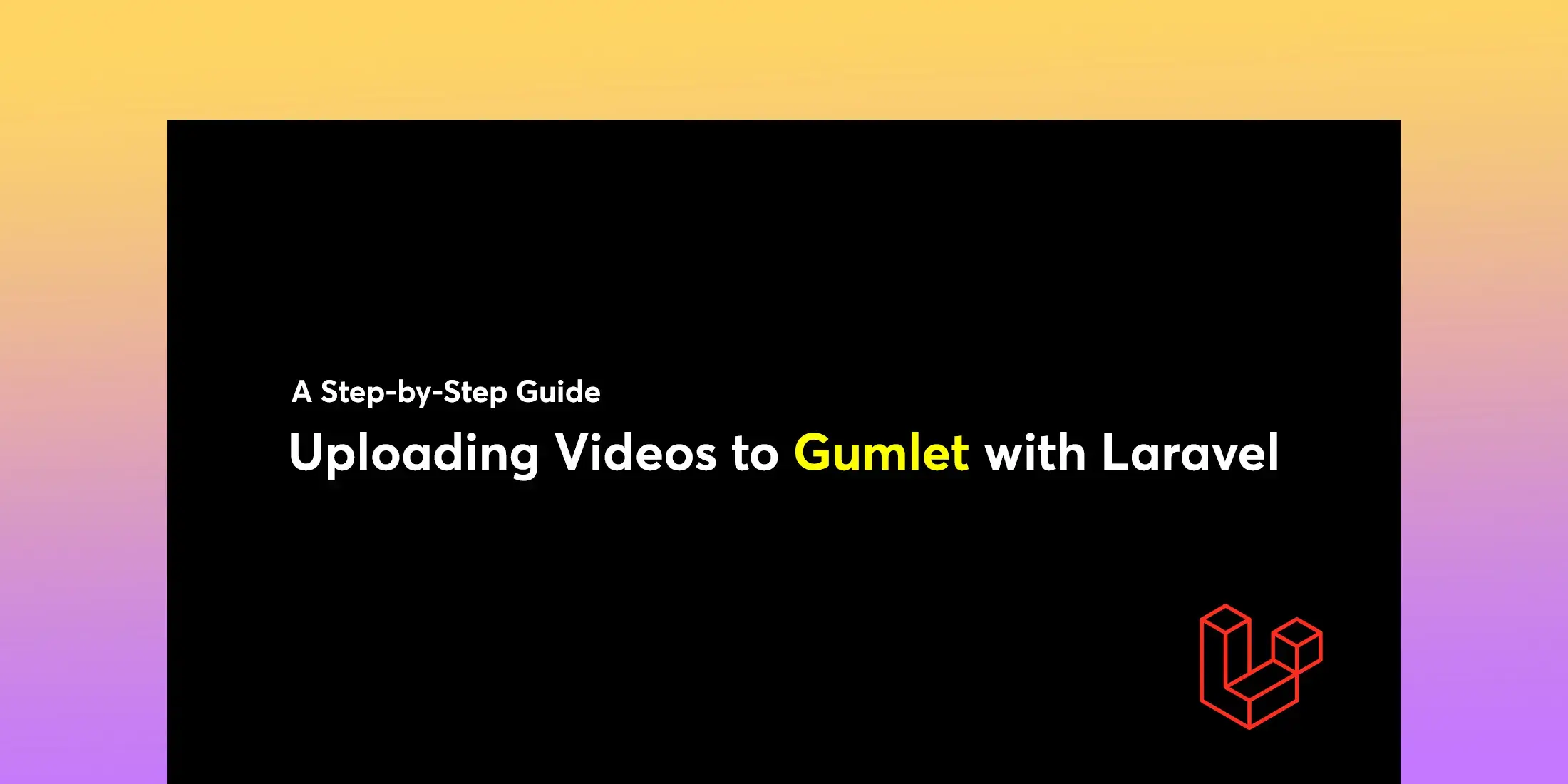
Uploading Videos to Gumlet with Laravel: A Step-by-Step Guide
In this article, we will learn how to upload videos to Gumlet in Laravel. Gumlet allows you to professionally host and embed videos and images on your website.We'll be using Laravel's HTTP client and Guzzle to interact with the Gumlet API for uploading and monitoring the status of video uploads.
Prerequisites
Before we begin, ensure that you have the following prerequisites in place:
Gumlet Account: You need a Gumlet account to get the API token and other necessary details.
Guzzle HTTP Client: Laravel comes with Guzzle, but if you don't have it installed, you can add it using Composer:
```php composer require guzzlehttp/guzzle ```Setting Up Environment Variables
First, we need to set up environment variables.
```php GUMLET_COLLECTION_ID= GUMLET_PROFILE_ID= GUMLET_API_TOKEN= ```  Creating the Video Upload Function
Create a function in your controller uploadGamlet, which upload videos to Gumlet.
```php public function uploadGumlet(Request $request) { $client = new Client(); $body = [ "format" => 'MP4', "input" => $request->video, "collection_id" => env('GUMLET_COLLECTION_ID'), "profile_id" => env('GUMLET_PROFILE_ID'), "title" => 'testing mp4 video' ]; $response = $client->request('POST', 'https://api.gumlet.com/v1/video/assets', [ 'body' => json_encode($body), 'headers' => [ 'Authorization' => 'Bearer ' . env('GUMLET_API_TOKEN'), 'accept' => 'application/json', 'content-type' => 'application/json', ], ]); if ($response->getBody()) { $responseOutput = json_decode($response->getBody(), true); dd($responseOutput); } return response()->json([ 'success' => true, 'message' => 'Video uploaded.', ]); } ```We have used laravel dd() helper function to see what we are getting in response after the success request.
Now if you want to see the progress, then we can use progress which is coming in a request.We will hit GET request to check the progress of a video.
```php if ($response->getBody()) { $responseOutput = json_decode($response->getBody(), true); $videoDetailData = [ 'status' => 'uploading_gumlet', "asset_id" => $responseOutput['asset_id'], "source_id" => $responseOutput['source_id'], "playback_url" => $responseOutput['output']['playback_url'], "thumbnail_url" => $responseOutput['output']['thumbnail_url'], "status_url" => $responseOutput['output']['status_url'], ]; $video = Video::create($videoDetailData); CheckVideoProgress::dispatch($video->id); } ```Monitoring Video Upload Progress
To ensure that the video upload is completed, we need to monitor the upload progress. Create a new funtion checkProgress which will check the progress of a video and print Log in storage/logs/laravel.log when it is completed 100%.
```php class CheckVideoProgress implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; public $videoDetailid; public $asset_id; public function __construct(int $videoid) { $videoDetail = Video::findOrFail($videoid); $this->videoDetailid = $videoid; $this->asset_id = $videoDetail->asset_id; } public function handle() { $output = $this->apiCall(); if ($output['progress'] === 100) { $video = Video::findOrFail($this->videoDetailid); $video->update(['status' => 'completed']); Log::info("completed"); } else { self::dispatch($this->videoDetailid)->delay(now()->addMinutes(2)); } } public function apiCall() { $client = new Client(); $response = $client->request('GET', 'https://api.gumlet.com/v1/video/assets/' . $this->asset_id, [ 'headers' => [ 'Authorization' => 'Bearer ' . env('GUMLET_API_TOKEN'), 'accept' => 'application/json', 'content-type' => 'application/json', ], ]); $responseOutput = json_decode($response->getBody(), true); return $responseOutput; } ```Explanation
Guzzle Client: We create a new instance of the Guzzle HTTP client.
Preparing the Request Body: The $body array contains the required parameters for the API request, such as the format, input URL, collection ID, etc.
Making the API Request: We send a POST request to the Gumlet API endpoint with the prepared body and headers.
Processing the Response: If the response contains a body, we decode the JSON response to get the video details such as status URL.
Checking Upload Progress: We call the checkProgress method with the status URL to monitor the progress of the video upload.
Creating Route
Now, we need to create a route to handle the video upload request.
```php Route::post('/upload-gumlet', [GumletController::class, 'uploadGumlet']); ```Creating View
Create a simple HTML form to allow users to upload videos.
```php ```