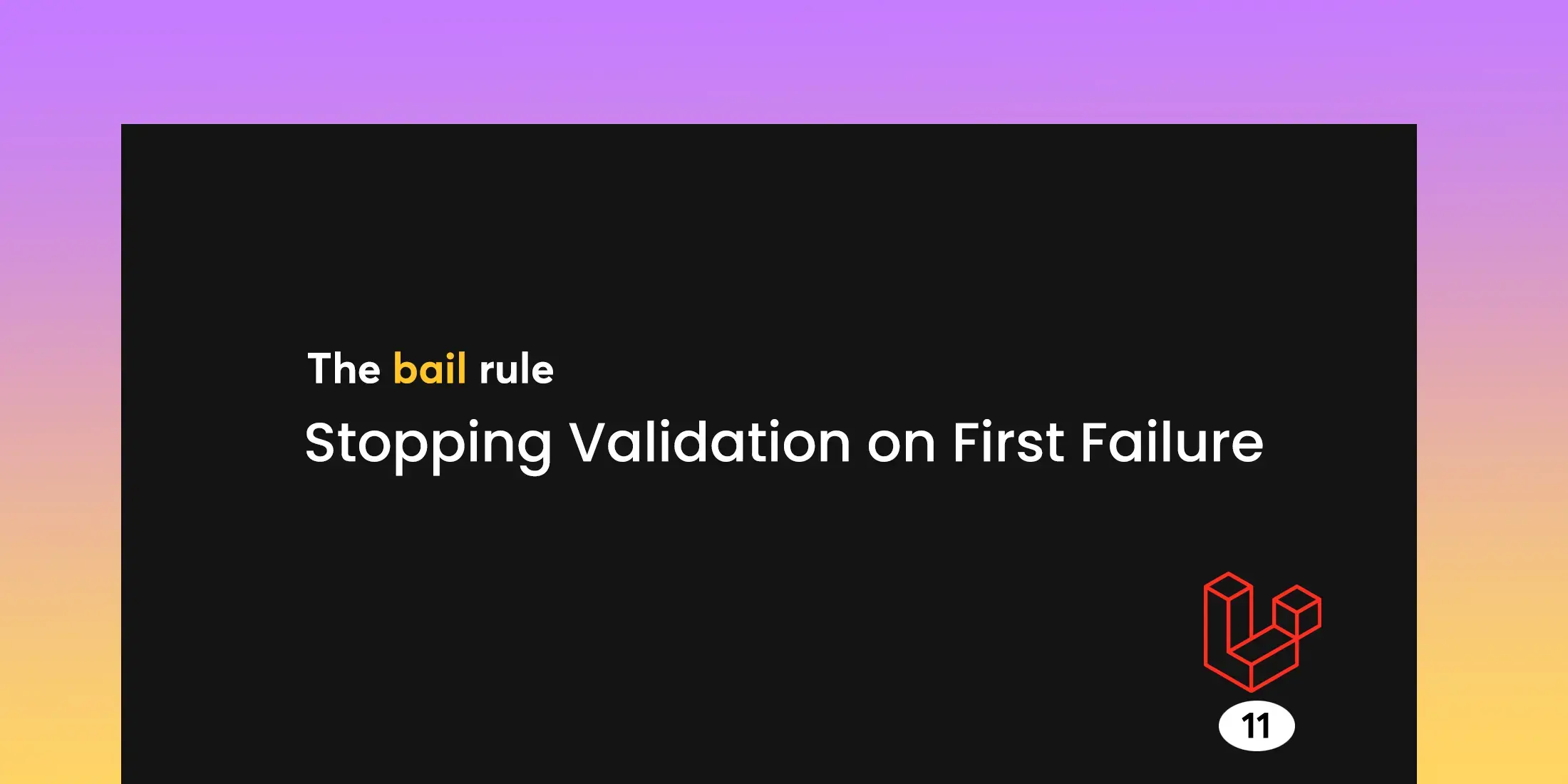
Laravel 11 Stopping Validation on First Failure
In Laravel, sometimes there is a scenario where you might want to stop further validation for an attribute as the first rule fails. This can be achieved in Laravel 11 using the bail rule.
The bail rule instructs Laravel to stop evaluating subsequent validation rules for an attribute if the current rule fails.
This can be particularly useful when you want to avoid unnecessary checks and improve performance.
Reference : Laravel Docs
Example 1
If the email format validation fails, Laravel will not proceed to check if the email is unique. This prevents unnecessary database queries and improves the efficiency of the validation process.
Advantages of Using bail
Performance: Reduces the number of validation rules processed, which can be beneficial for performance, especially with complex rules.
Clarity: Simplifies validation logic by ensuring that only the necessary rules are applied based on the outcome of the first rule.
Example 2
If you have created a separate request class for validation, you can also achieve it by adding stopOnFirstFailure attribute in class.