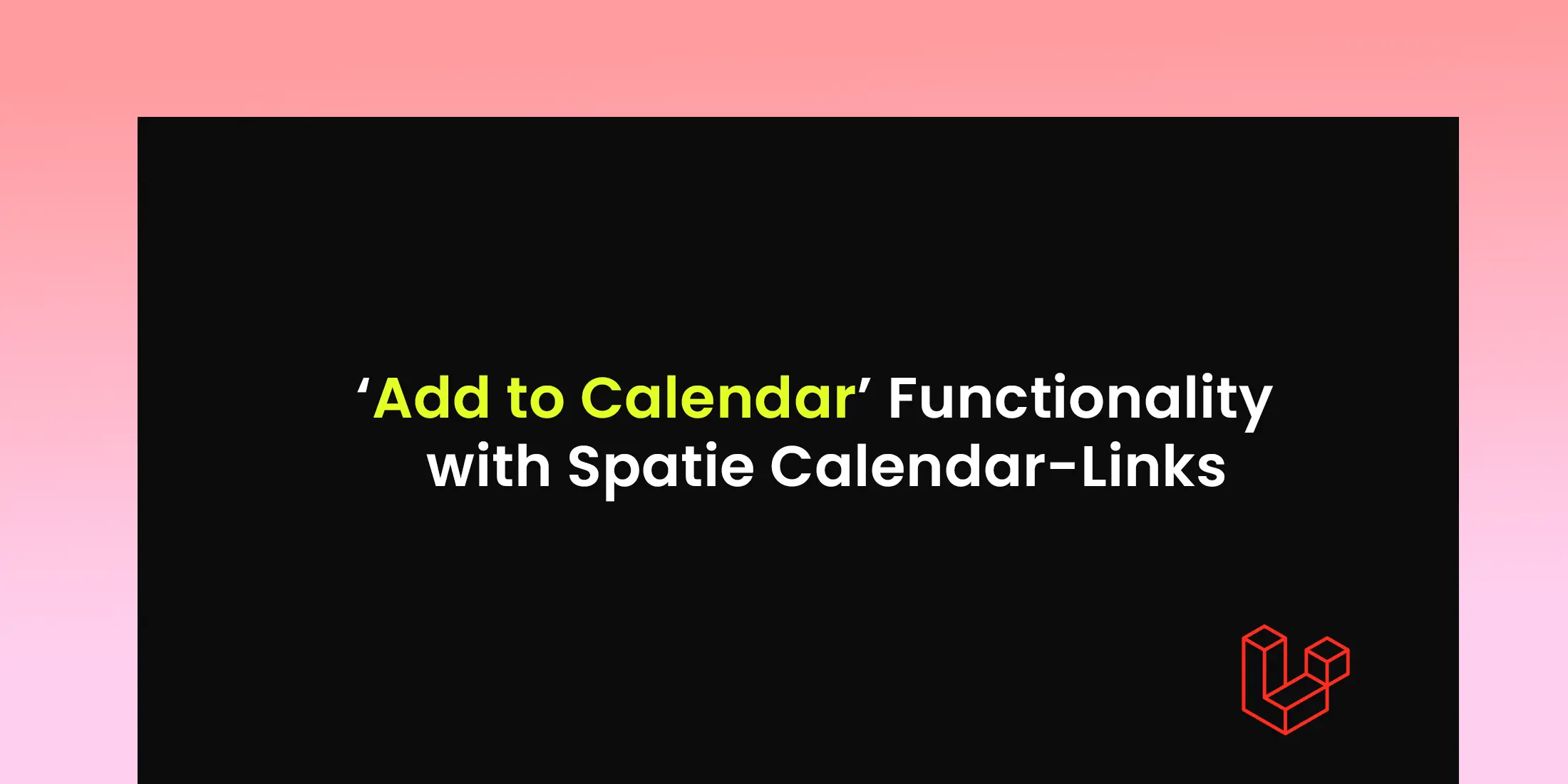
How to Easily Add ‘Add to Calendar’ Functionality in Laravel with Spatie Calendar-Links
Imagine this: You’ve built a fantastic Laravel application that includes upcoming events, meetings, or deadlines, and now you want to make it easier for users to add these events to their preferred calendars. Think of the convenience! With a single click, they can save your event to Google Calendar, Outlook, or iCal, making sure they don’t miss out on anything important. That’s exactly what Spatie’s Calendar-Links package is designed for.
Integrating "Add to Calendar" functionality into your Laravel application allows users to easily add events to their preferred calendar systems. The spatie/calendar-links package simplifies this process by providing a straightforward way to generate links for various calendar platforms like Google Calendar, iCal, and Outlook.
In this tutorial, we will learn how to use spatie/calendar-links in Laravel to generate these calendar links, including installation, basic usage, and advanced features.
Step 1: Installing the Package
We need to install spatie/calendar-links via Composer. Open your terminal and run:
composer require spatie/calendar-links
This command will add the spatie/calendar-links package to your Laravel project.
Step 2: Generating Calendar Links
Let’s set up a sample event and generate calendar links for it using the Link::create method.
Imagine we have a team meeting scheduled, and we want to make sure it’s added to everyone’s calendar. Here’s how we’d go about it:
Define the event details: We’ll start by defining the event title, description, location, start time, and end time.
Create the calendar links: Using the Link::create method, we’ll generate URLs for Google Calendar, iCal, and Outlook.The spatie/calendar-links package is used to provide API for generating calendar links.
// Define event details
$eventTitle = 'Meeting with Team';
$eventDescription = 'Discuss project milestones and deadlines.';
$eventLocation = 'Conference Room 1';
$eventStartTime = new \DateTime('2024-11-2 10:00:00');
$eventEndTime = new \DateTime('2024-11-4 11:00:00');
// Create calendar links
$googleCalendarLink = Link::create($eventTitle, $eventStartTime, $eventEndTime)
->description($eventDescription)
->address($eventLocation)
->google();
$icalLink = Link::create($eventTitle, $eventStartTime, $eventEndTime)
->description($eventDescription)
->address($eventLocation)
->yahoo();
$outlookLink = Link::create($eventTitle, $eventStartTime, $eventEndTime)
->description($eventDescription)
->address($eventLocation)
->webOutlook();
In the above example, we define the event details and use the Link::create method to generate links for Google Calendar, iCal, and Outlook. Each method (google, ical, outlook) returns a URL that can be used to add the event to the respective calendar system.
Step 3: Integrating with Laravel Views
<!DOCTYPE html>
<html>
<head>
<title>Add to Calendar - Elegant Laravel</title>
</head>
<body>
<h3>Add to Calendar</h3>
<ul>
<li><a href="{{ $googleCalendarLink }}" target="_blank">Add to Google Calendar</a></li>
<li><a href="{{ $icalLink }}" target="_blank">Add to iCal</a></li>
<li><a href="{{ $outlookLink }}" target="_blank">Add to Outlook Calendar</a></li>
</ul>
</body>
</html>
In the above example, the controller generates calendar links and passes them to the view. The Blade template then displays these links, allowing users to add the event to their preferred calendar system.
Setting Up Event Details with Spatie’s Calendar-Links
Let’s set up two sample events:
Usama's Birthday – A casual celebration.
Team Meeting – A business event with more details, such as organizer, attendees, meeting links, attachments, and reminders.
Usama's Birthday Event
Here’s a straightforward event setup with a start and end time, description, and address for Usama's birthday party.
<?php
use Spatie\CalendarLinks\Link;
use DateTime;
// Define the event start and end times
$from = DateTime::createFromFormat('Y-m-d H:i', '2018-02-01 09:00');
$to = DateTime::createFromFormat('Y-m-d H:i', '2018-02-01 18:00');
// Create the event link
$link = Link::create('Usama Birthday', $from, $to)
->description('Cookies & cocktails!')
->address('123 Street, ABC Country');
// Generate the calendar links
echo $link->webOffice();
echo $link->ics();
Here, we’ve defined a simple event with a title, time, and location. The webOffice() method generates a link to Outlook’s Office 365 calendar, and ics() generates an ICS data URI, which can be used for iCal and Outlook.
Adding Advanced Details for a Team Meeting
For the team meeting, let’s go deeper and add additional information such as the organizer, attendees, priority, status, and a meeting link. This makes the event comprehensive and professional.
<?php
use Spatie\CalendarLinks\Link;
use DateTime;
// Define event details
$eventTitle = "Meeting with Team";
$eventDescription = 'Discuss project milestones and deadlines.';
$eventLocation = 'Conference Room 1';
$eventStartTime = new DateTime('2024-11-02 10:00:00');
$eventEndTime = new DateTime('2024-11-02 11:00:00');
// Additional event details
$organizer = [
'name' => 'John Doe',
'email' => 'john.doe@example.com'
];
$attendees = [
['name' => 'Jane Smith', 'email' => 'jane.smith@example.com'],
['name' => 'Bob Johnson', 'email' => 'bob.johnson@example.com']
];
$eventDetails = [
'category' => 'Meeting',
'priority' => 'High',
'status' => 'Confirmed',
'recurring' => false,
'timezone' => 'America/New_York',
'reminder' => '30', // minutes before
'notes' => "Please bring your project updates.\nDon't forget to review the previous meeting notes.",
'meetingLink' => 'https://meet.example.com/abc123',
'attachments' => [
'agenda.pdf',
'previous_minutes.doc'
]
];
// Create the base calendar link
$baseLink = Link::create($eventTitle, $eventStartTime, $eventEndTime)
->description($eventDescription . "\n\n" .
"Organizer: {$organizer['name']}\n" .
"Meeting Link: {$eventDetails['meetingLink']}\n\n" .
"Notes:\n{$eventDetails['notes']}")
->address($eventLocation);
// Generate links for different calendar providers
$calendarLinks = [
'google' => $baseLink->google(),
'outlook' => $baseLink->webOutlook(),
'office365' => $baseLink->webOffice(),
'yahoo' => $baseLink->yahoo(),
'ics' => $baseLink->ics()
];
// Print the links to preview
dd($calendarLinks)
Explanation of Advanced Details
Organizer: Adding organizer information helps users know who scheduled the meeting.
Attendees: You can display attendees’ names and emails as additional context (e.g., in the event description).
Additional Fields: Details such as category, priority, status, and reminder can be used to indicate the importance and nature of the event.
Meeting Link and Attachments: By embedding a meeting link and listing attachments, you make it convenient for users to join the meeting or access documents without additional steps.
Applications of spatie/calendar-links
-
Event Management Systems: Allow users to easily add events to their calendars from an event management system.
-
Appointment Booking:Users can add their appointment to their personal calendar.
-
Email Notifications: Include calendar links in email notifications for events, meetings, or reminders.
Final Thoughts
With Spatie’s Calendar-Links package, you can easily transform a simple event into a comprehensive, well-documented event that users can add to their calendar. Whether you’re setting up a casual birthday party or coordinating a critical business meeting, this functionality ensures a smooth and complete experience for your users.
Feel free to give it a try and witness just how simple and seamless adding events to calendars can be! If you have any questions or need further assistance, don’t hesitate to leave a comment below. We’d love to help!