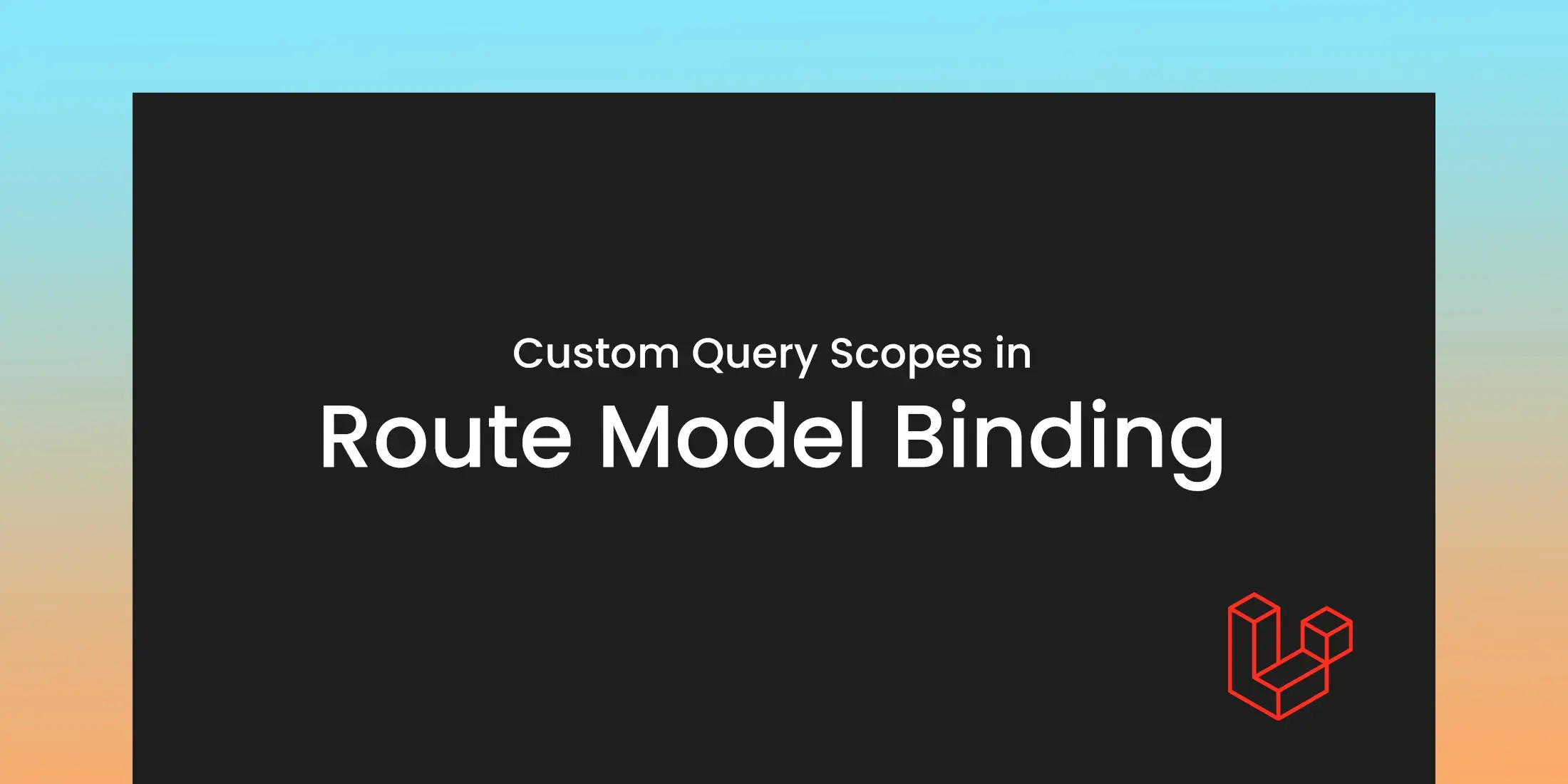
Laravel 11 - Custom Query Scopes in Route Model Binding
Laravel 11 introduces an exciting feature that allows developers to define custom query scopes for implicit model binding. This feature provides more flexibility and control over how models are retrieved based on route parameters.
Route model binding is a way to automatically fetch a model based on a route parameter. For example, if you have a route like /posts/{post}, where {post} represents a post ID, Laravel will retrieve the corresponding post model for you.
In Laravel 11, you can now specify custom query scopes for implicit model binding. This allows you to customize how models are fetched when using route model binding,
Example Implementation
Step 1: Define a Custom Scope
First, define a custom scope in your Post model to filter published posts:
use Illuminate\Database\Eloquent\Builder;
class Post extends Model
{
// Define a custom scope for route model binding
public function scopePublished(Builder $query)
{
return $query->where('is_published', true);
}
}
Step 2: Use the Custom Scope in Route Model Binding
In your web.php file, define a route that uses the custom scope:
Route::get('/posts/{post:slug}', function (Post $post) {
return view('post.show', compact('post'));
})->scopeBindings('published');
In this route definition, the scopeBindings method is used to apply the published scope when fetching the Post model. The {post:slug} parameter indicates that the post should be retrieved by its slug instead of its ID.
Benefits of Custom Query Scopes
Increased Flexibility: Custom query scopes allow you to define specific criteria for retrieving models.
Cleaner Code: By defining scopes in your models, you can keep your routes and controllers clean and focused.
Reusability: Custom query scopes can be reused across different parts of your application.
Overall, this enhancement in Laravel 11 allows you to customize the retrieval of models during route model binding by using custom query scopes. It gives you more flexibility and control over how models are fetched based on the route parameters.