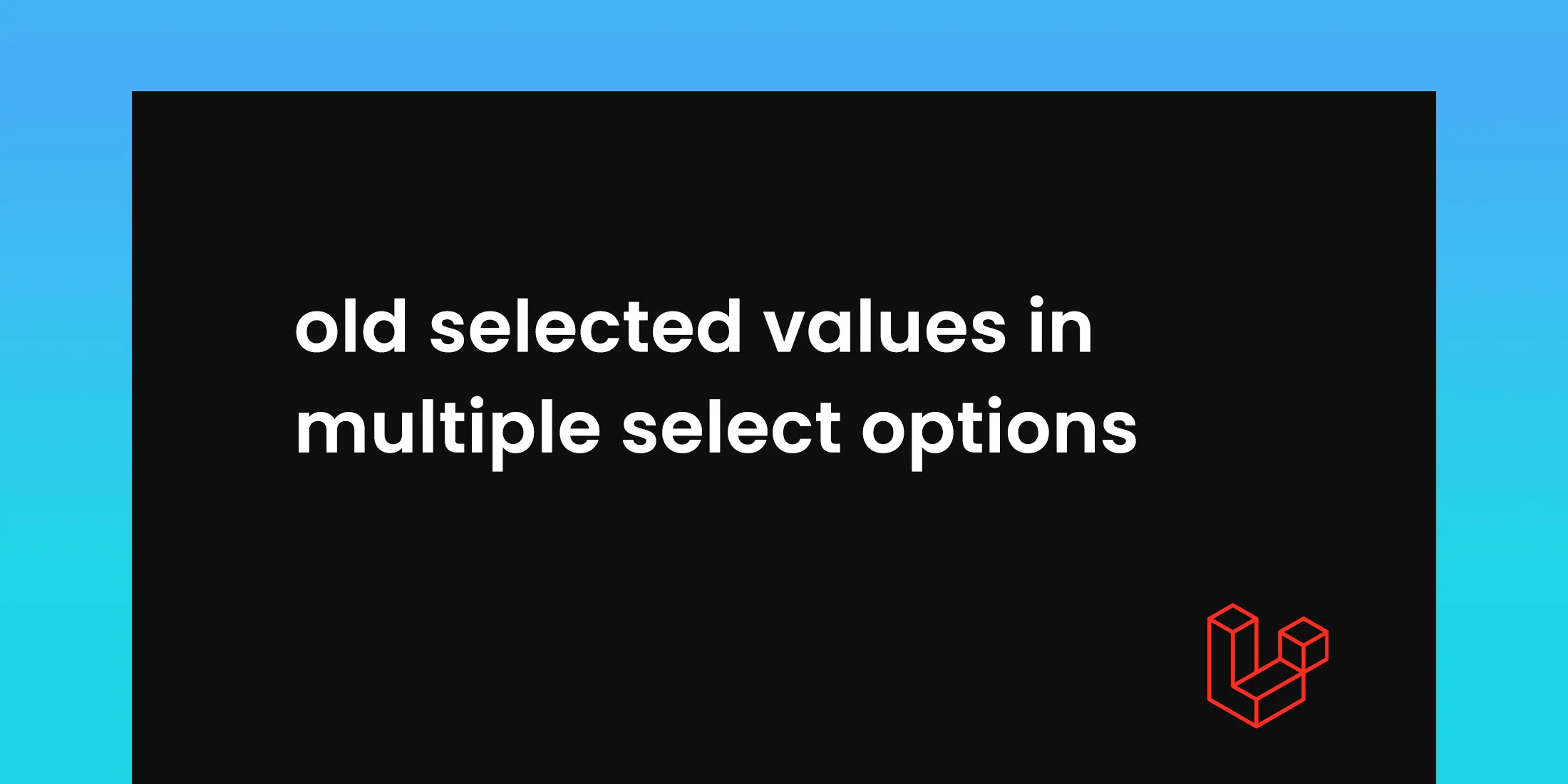
How to show old selected values in multiple select options in Laravel
Explanation of the Code
Label and Select Element
<label>: The label is tied to the select box via the for
attribute, improving accessibility. It’s crucial for screen readers and a better user experience.
<select>: The multiple
attribute allows multiple categories to be selected. The name
attribute is set to category_id[]
to handle multiple selections properly when the form is submitted.
Rendering the Categories:
@foreach ($categories as $category): This loop iterates over all available categories, generating an <option>
element for each.
<option value="{{ $category->id }}">: The value
attribute is set to the category’s ID. This is necessary for submitting the selected categories back to the server.
{{ in_array($category->id, $post?->categories->pluck('id')->toArray() ?? []) ? 'selected' : '' }}
: This statement checks if the current category ID exists in the list of categories associated with the post.
- nullsafe operator (post?): Safely accesses
$post->categories
even if the$post
object is null (e.g., when creating a new post). - pluck('id')->toArray(): Extracts the category IDs associated with the post and converts them into an array.
- in_array(): Checks if the current category ID is part of the selected categories. If it is, the
selected
attribute is added, which pre-selects the category in the dropdown.
Benefits of This Approach
- Clarity: Clearly shows which categories are selected based on the post object.
- Flexibility: Handles both creating and updating posts with selected categories.
- Error Display: Properly shows validation errors related to category selection.