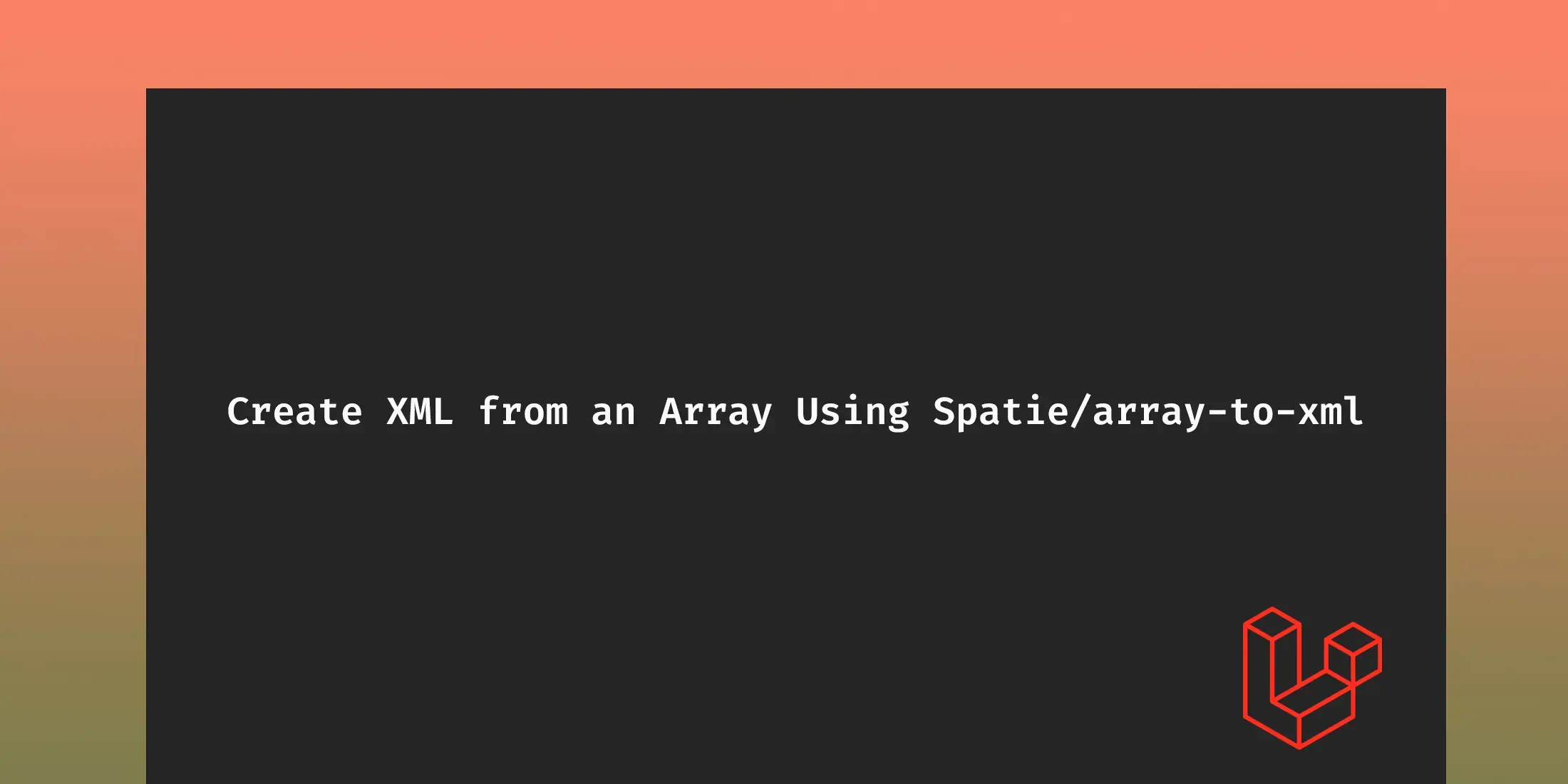
How to Create XML from an Array Using Spatie(array-to-xml) in Laravel
To converting PHP arrays into XML documents the spatie/array-to-xml package is used.This functionality is useful when working with systems that require XML data, such as third-party APIs or legacy systems. We will explain step by step the installation, basic usage, advanced customization, and real-world applications of spatie/array-to-xml package in a Laravel environment.
1. Installing the Package
To use the spatie/array-to-xml package, install it with the help of Composer by using the following code:
composer require spatie/array-to-xml
The above code dds the package to your Laravel project, allowing you to use its XML conversion capabilities.
2. Basic Usage
Once spatie/array-to-xmlpackage is installedit allows for conversion of arrays to XML. Given below is the code for a basic example:
$array = [
'user' => [
'name' => 'John Doe',
'email' => 'john@example.com',
],
'articles' => [
[
'title' => 'First Article',
'content' => 'This is the content of the first article.',
],
[
'title' => 'Second Article',
'content' => 'This is the content of the second article.',
],
],
];
$xml = ArrayToXml::convert($array);
echo $xml;
The above code will give the following output:
<root>
<user>
<name>John Doe</name>
<email>john@example.com</email>
</user>
<articles>
<item>
<title>First Article</title>
<content>This is the content of the first article.</content>
</item>
<item>
<title>Second Article</title>
<content>This is the content of the second article.</content>
</item>
</articles>
</root>
The ArrayToXml::convert method converts a PHP array into an XML string, using the default root element name root.
3. Customizing the XML Output
The package gives you options for customization of the XML output. You can specify different root element names, add attributes, and manage empty values. Given Below is the code for the example:
use Spatie\ArrayToXml\ArrayToXml;
$array = [
'user' => [
'_attributes' => ['id' => 1],
'name' => 'John Doe',
'email' => 'john@example.com',
],
'articles' => [
[
'_attributes' => ['type' => 'tech'],
'title' => 'First Article',
'content' => 'This is the content of the first article.',
],
[
'_attributes' => ['type' => 'news'],
'title' => 'Second Article',
'content' => 'This is the content of the second article.',
],
],
];
$xml = ArrayToXml::convert($array, 'userData');
echo $xml
The above code will give the following output:
<userData>
<user id="1">
<name>John Doe</name>
<email>john@example.com</email>
</user>
<articles>
<item type="tech">
<title>First Article</title>
<content>This is the content of the first article.</content>
</item>
<item type="news">
<title>Second Article</title>
<content>This is the content of the second article.</content>
</item>
</articles>
</userData>
In the above example, the root element is changed to userData, and attributes are added to elements.
4. Handling Special Cases
The package handles various edge cases:
Empty Values: By default, empty values are not included in the XML. If needed, you can include them using the following code:
use Spatie\ArrayToXml\ArrayToXml;
$array = [
'user' => [
'name' => 'John Doe',
'email' => null,
],
];
$xml = ArrayToXml::convert($array, 'user', true, 'UTF-8', '1.0', ['emptyElementSuffix' => '_empty']);
echo $xml;
The above code will give the following output:
<user>
<name>John Doe</name>
<email_empty />
</user>
Special Characters: Special characters like <, >, and & are automatically escaped to maintain valid XML. For example when using the following code:
$array = [
'user' => [
'name' => 'John & Jane Doe',
],
];
$xml = ArrayToXml::convert($array);
echo $xml;
The output will be:
<root>
<user>
<name>John & Jane Doe</name>
</user>
</root>
5. Real-World Use Cases
The ability to convert arrays to XML is invaluable in several scenarios:
API Integrations: When working with third-party APIs that require XML data, converting arrays to XML ensures smooth integration.
Legacy Systems: Many legacy systems use XML for data interchange. This package helps in bridging modern PHP applications with such systems.
Data Exports: XML is often used for exporting data in a structured format. This package facilitates exporting data from Laravel applications.
6. Comparisons with Other XML Packages
While spatie/array-to-xml is powerful and easy to use, there are other packages available for XML conversion. For example:
SimpleXML: A built-in PHP extension that provides a simple API for XML parsing and generation. It offers less control over XML output compared to spatie/array-to-xml.
XMLWriter: A PHP extension for creating XML documents. It provides more control over XML formatting but requires more effort to use.
spatie/array-to-xml is favored for:
- its ease of use
- flexibility
- ability to work smoothly with PHP arrays.
7. Integration with Other Laravel Features
The spatie/array-to-xml package integrates well with other Laravel features:
Controllers: Use the package in Laravel controllers to return XML responses for API endpoints.
Jobs and Queues: Convert data to XML within queued jobs for processing and exporting.
Scheduled Tasks: Automate XML data exports using Laravel'stask scheduling.