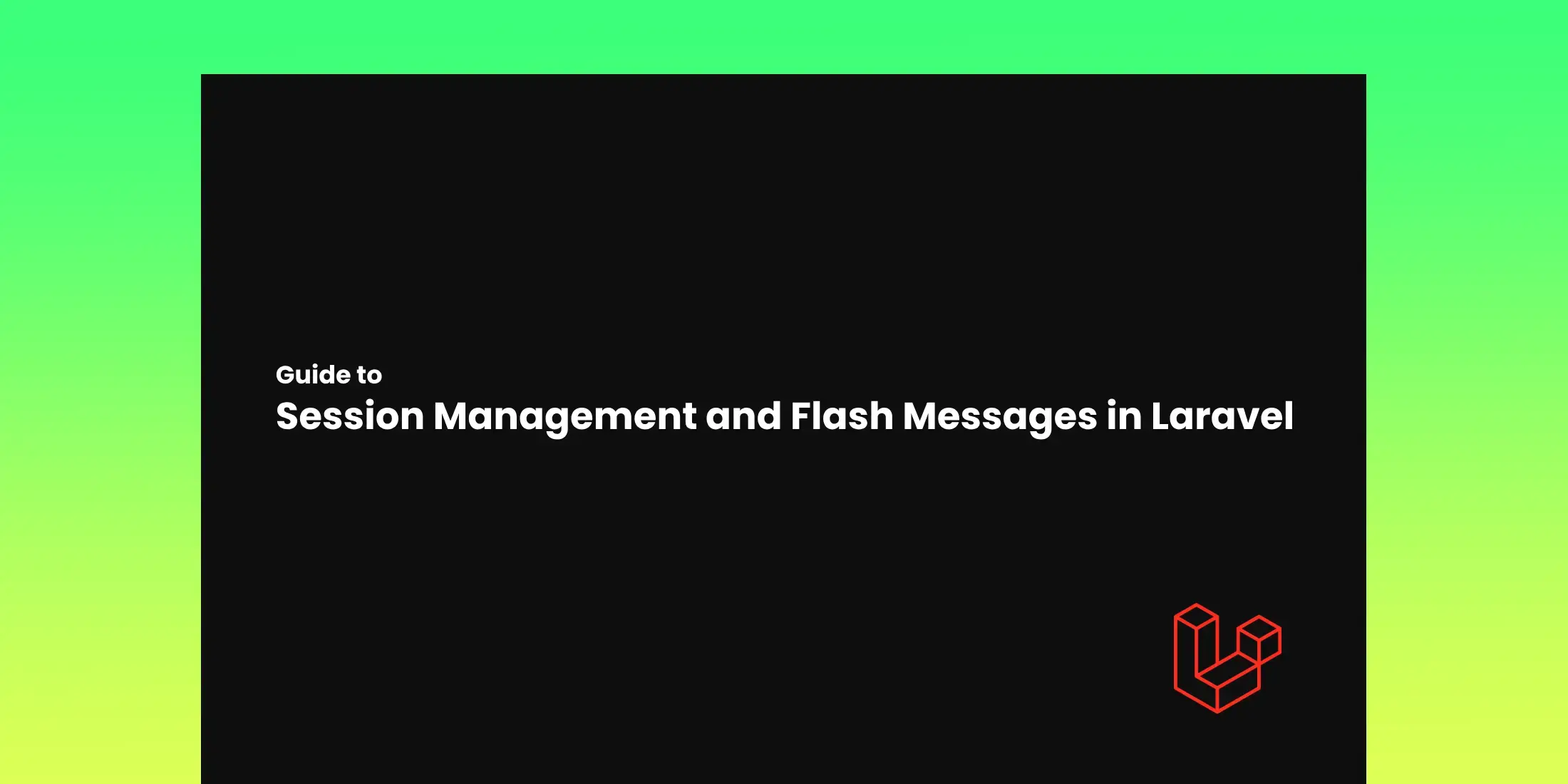
Guide to Session Management and Flash Messages in Laravel
Laravel, one of the most popular PHP frameworks, offers various features that make web development smooth and efficient. Among these features, session management and flash messages play a crucial role in handling user interactions and delivering feedback.
What is Session Management in Laravel?
Sessions in Laravel provide a way to store data across multiple requests for a user. This data is often used to track users as they move through the application, maintaining user preferences, login states, or even temporary data like shopping cart items. Laravel's session management is built on top of PHP’s native session handling but offers more flexibility with its diverse drivers, allowing you to store session data in various ways.
Key Components of Laravel Session Management
Session Configuration:
Laravel’s session configuration file is located in config/session.php. This file defines various session settings such as the session driver, session lifetime, and security options.
Session Drivers:
Laravel session drivers, which dictate where session data is stored. These include:
- file (stores sessions in storage/framework/sessions)
- cookie (stores sessions in browser cookies)
- database (stores sessions in a database table)
- redis, memcached, and dynamodb (used for high-performance session handling)
- array (used for testing; sessions are stored in memory and not persisted)
Session Lifetime:
You can control how long sessions should be kept alive before they expire:
'lifetime' => 120, // in minutes
This value ensures that sessions are automatically cleared after a specific period of inactivity.
Session Security:
Laravel includes built-in security features for sessions. The encrypt setting ensures session data is encrypted before it is stored, and the secure option ensures that session cookies are only sent over HTTPS connections.
How to Use Sessions in Laravel
Storing Data in the Session
Storing data in Laravel's session is easy. You can do so using the session helper:
This method stores the given key-value pair in the session. The session persists across multiple requests, making it ideal for storing temporary data such as user login states or preferences.
Retrieving Session Data
To retrieve session data, you can use the same session helper:
If the key does not exist, Laravel will return null by default. You can also pass a second argument to provide a default value if the key is not found:
Checking if Data Exists in the Session
To check whether a specific session key exists:
Removing Data from the Session
To remove an item from the session:
If you want to clear all session data:
What are Flash Messages?
Flash messages are a type of session data in Laravel that only persist for one request. They are commonly used to send short-term user feedback, like success or error messages, after form submissions, login attempts, or actions requiring feedback. Once displayed, they are automatically cleared in the next request.
For example, when a user successfully submits a form, you might want to display a message like "Your form was submitted successfully!" before the next page loads or if the form was not submitted, you might want to display a message like "Error form was not submitted please try again".
Why Use Flash Messages?
Flash messages enhance user experience by providing quick feedback on user actions without cluttering the interface. They are a common feature in web applications, used for tasks such as:
- Showing a "Login successful" message.
- Displaying validation errors after form submissions.
- Notifying users about changes in their profile or settings.
How to Use Flash Messages in Laravel
Flashing Session Data
Laravel has a flash method flash method stores data in the session only for the next request. Here's how to flash a message:
After flashing the message, you can retrieve and display it in your views. For example, in your Blade template:
Flashing Data with Redirects
Flash messages are often used with combination with redirects. After a form is submitted or a certain action is performed, you typically redirect the user to another page and flash a message in the process:
In this case, the with method allows you to flash data to the session while performing a redirect. The message can then be retrieved on the redirected page using the session helper as shown above.
Flashing Multiple Messages
Laravel also allows you to flash multiple pieces of data to the session in a single request:
Then, you can retrieve both values in your view:
Keeping Flash Messages for Multiple Requests
By default, flash messages only persist for the next request. However, you can instruct Laravel to keep the message for additional requests by using the reflash method:
If you only want to keep specific flash data, use the keep method:
Displaying Flash Messages in Blade Views
In Laravel, the most common place to display flash messages is in Blade views. You can conditionally display a flash message by checking if the session contains specific data:
This snippet can be added to your master layout, ensuring that flash messages are displayed across the application.
Using Middleware to Handle Flash Messages
In some cases, you may want to handle flash messages globally across your application. You can do this using Laravel middleware. For example, you can create a middleware to ensure flash messages are handled properly after every form submission or user action.
Create a middleware using the Artisan command:
In handle method of the middleware, we can implement logic to manage flash messages:
Now we just have to apply the middleware in app/Http/Kernel.php and use it when you that need flash Messages.
Advanced Session Management in Laravel
Storing Complex Data in Sessions
While sessions are typically used to store simple key-value pairs, you can also store more complex data such as arrays and objects:
Database Session Driver
Laravel’s database session driver allows you to store session data in a database. This is useful for large-scale applications where performance and data persistence are critical.
First, create the sessions table using the Artisan command:
Next, update the session driver in config/session.php:
Once this is set up, Laravel will store session data in the database instead of files or cookies.
Best Practices for Session Management and Flash Messages
Use Flash Messages for Temporary Feedback:
Flash messages should be used to provide quick feedback on user actions, such as form submissions or logins. Do not store critical information in flash messages, as they are automatically deleted after one request.
Keep Sessions Secure:
Always enable encryption for session data in production environments to protect sensitive information. Also, use secure cookies (secure option) to ensure that session data is transmitted securely over HTTPS.
Limit Session Data:
Avoid storing large datasets or sensitive information like passwords in sessions. Use sessions sparingly and only for necessary data like user states or preferences.
Clear Session Data When No Longer Needed:
Use session()->forget('key') or session()->flush() to remove data that is no longer required. This helps prevent sessions from becoming bloated and keeps the user experience smooth.