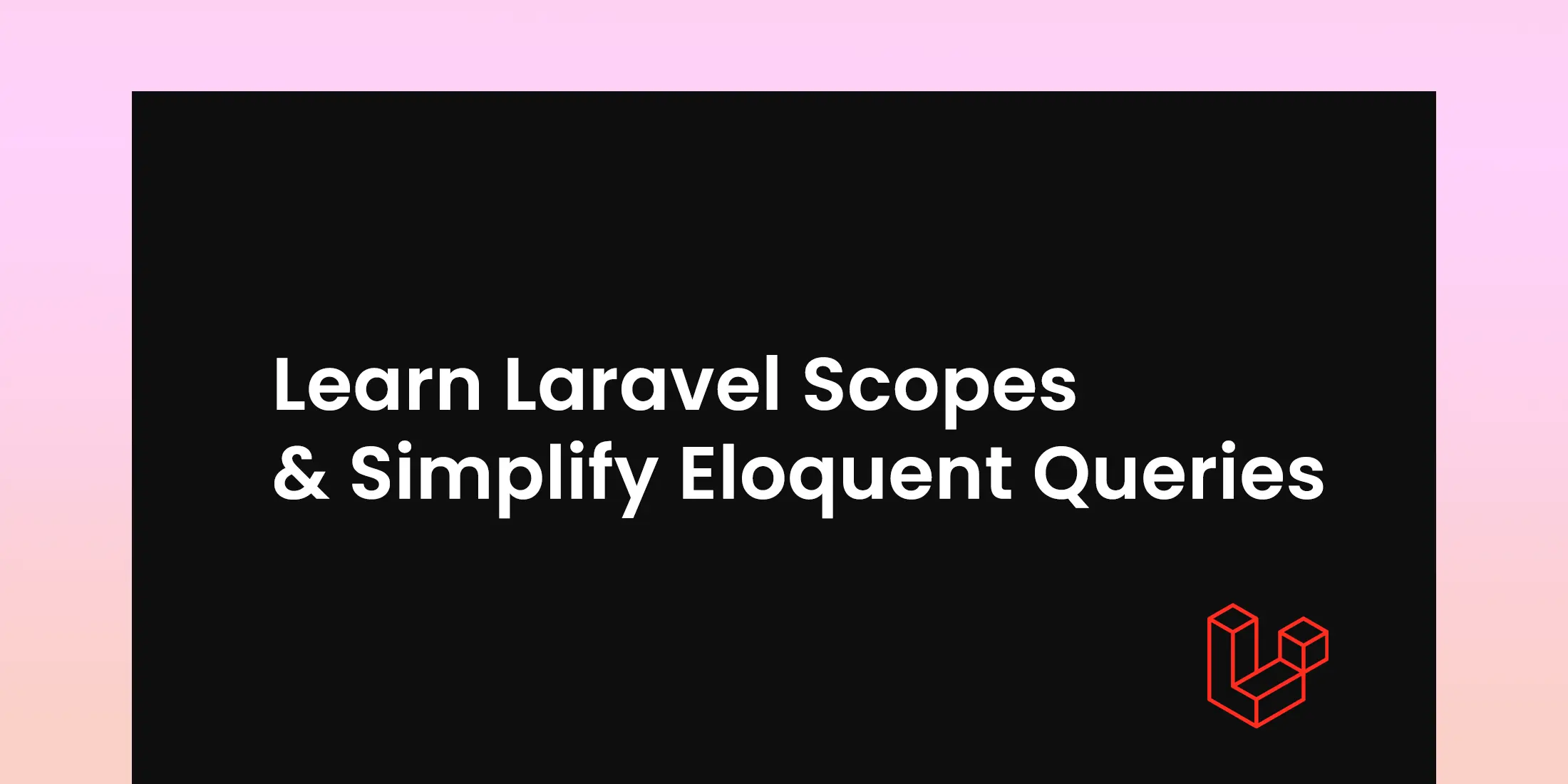
Learn Laravel Scopes and simplify Eloquent Queries
In this article we learn how to use Laravel scopes from scratch which you can reuse in a query and you don’t need to duplicate . Scopes in laravel allow you to define reusable query constraints that can be applied to queries on your models.It made code more organized, readable.We have two types of scopes , Local Scope and Global Scope.
Creating a Scope (Local Scope)
You can define a scope by adding a method to your Eloquent model. The method name should start with scope, followed by a meaningful name describing the scope's purpose.
class Post extends Model
{
// Scope to retrieve only published posts
public function scopePublished($query)
{
return $query->where('published', true);
}
}
Using a Scope
We can use it like any other Eloquent method when querying your model.
$publishedPosts = Post::published()->get();
Scopes can also accept parameters, allowing for dynamic behavior.
public function scopeStatus($query, $status)
{
return $query->where('status', $status);
}
$draftPosts = Post::status('draft')->get();
Global Scopes
Global scopes automatically apply to all queries for a given model.
public static function booted()
{
static::addGlobalScope('published', function ($query) {
$query->where('published', true);
});
}
Removing Scopes
if you need to temporarily remove a scope,this is how you can do that.
$posts = Post::withoutGlobalScope('published')->get();