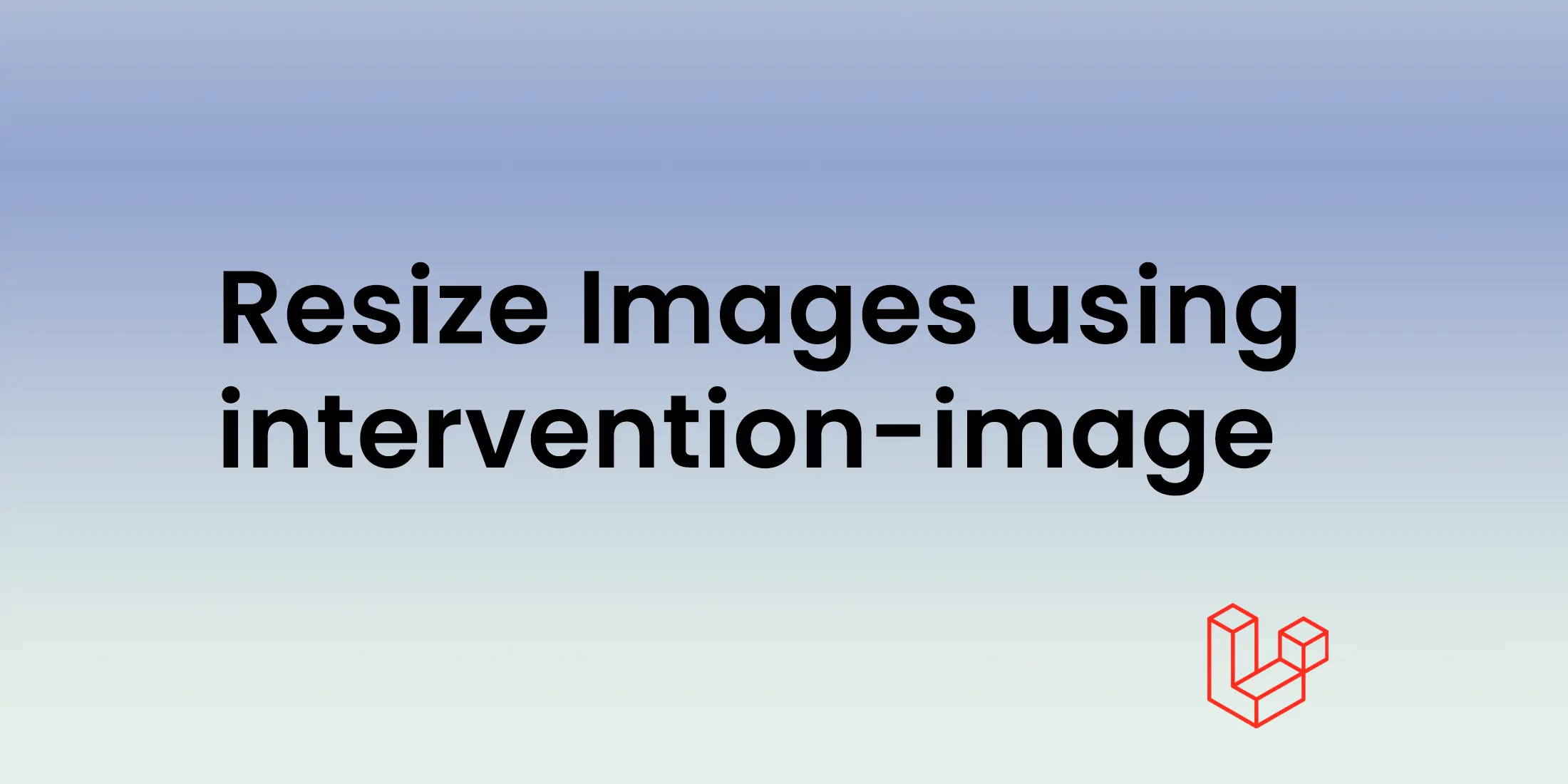
Resize Images using intervention-image
In this article we will learn how to install and use intervention/image in laravel.We will resize images using intervention/image package.We will upload multiple images and add a check if a image size is greater than 25mb we will resize it.
Step 1: Install the Intervention/Image Package
To get started, install the intervention/image package using Composer:
composer require intervention/image-laravel
Next, add the configuration files to your application using the vendor:publish command:
php artisan vendor:publish --provider="Intervention\Image\Laravel\ServiceProvider"
Step 2: Create a View for Image Upload
Create a view file (e.g., resources/views/upload.blade.php) and add a form to upload multiple images:
<form action="{{ route('upload.images') }}" method="post" enctype="multipart/form-data">
@csrf
<input type="file" id="images" name="images[]" multiple>
<button class="btn btn-primary" type="submit">Upload</button>
</form>
Step 3: Define the Route
In your routes/web.php file, define the route for the form action:
use App\Http\Controllers\ImageUploadController;
Route::post('/upload-images', [ImageUploadController::class, 'saveImages'])->name('upload.images');
Step 4: Implement the Controller Logic
Create a controller (e.g., ImageUploadController.php) and implement the logic to handle the image upload and resizing:
Now in our controller we will write our main business logic.
public function saveImages(Request $request)
{
// Validate the request
$request->validate([
'images' => 'required|array',
'images.*' => 'required|image|mimes:jpeg|max:25600' //max-size 25mb
]);
try {
if ($request->has('images')) {
$images = $request->file('images');
$folderName = now()->format('Y-m-d');
$destinationPath = public_path('images/' . $folderName);
// Create directory if it doesn't exist
if (!File::isDirectory($destinationPath)) {
File::makeDirectory($destinationPath, 0777, true, true);
}
foreach ($images as $image) {
$img = Image::read($image);
// Check if the image exceeds 25 megapixels
if ($img->width() * $img->height() > 25 * 1000000) {
// Resize the image
$img->resize(5000, null, function ($constraint) {
$constraint->aspectRatio();
});
}
$imageName = time() . '-' . $image->getClientOriginalName();
$img->save($destinationPath . $imageName);
}
return back()->with('success', 'Images Upload Successfully.');
}
} catch (\Exception $e) {
return $e->getMessage();
}
}
Explanation
Validation: Ensure the request contains an array of images and validate each image's type and size.
File Handling: Check if the request has images.
Directory Creation: Create a directory with the current date if it doesn't already exist.
Image Processing: Iterate through each image, create an instance using Image::make, and check its size. If the image size exceeds 25MB, resize it to a width of 5000 pixels while maintaining the aspect ratio.
Save Image: Save the processed image to the specified directory with a unique name.
Return Response: Return a success message if the images are uploaded successfully or an error message if an exception occurs.