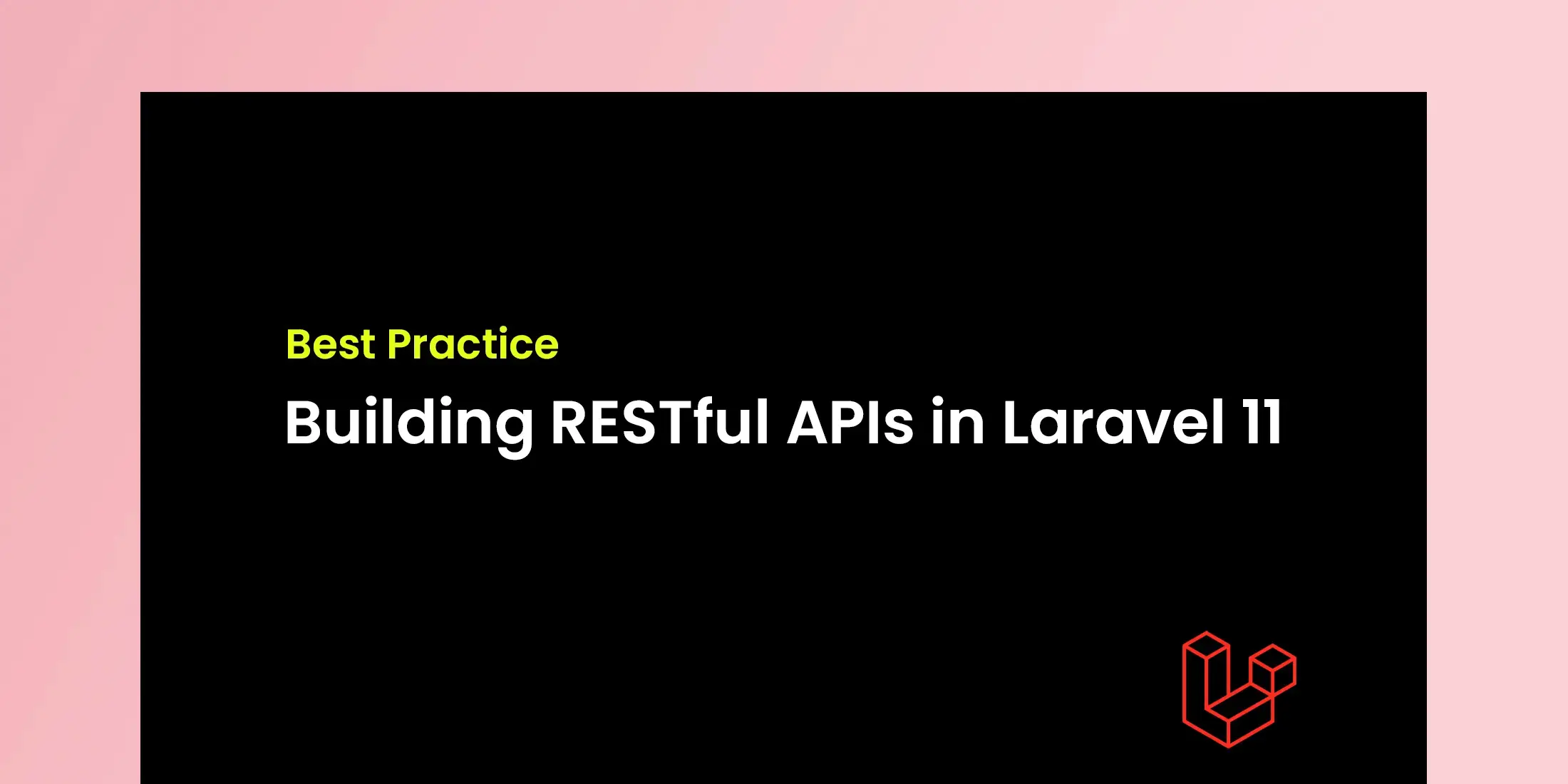
Building RESTful APIs in Laravel 11: A Simple Guide
Building RESTful APIsin Laravel is useful for developers who need to expose their application's data to other platforms or mobile devices. Laravel provides powerful tools to design APIs that follow REST principles, ensuring a clean, maintainable, and scalable architecture. In this tutorial, we will explore how to build RESTful APIs with Laravel and the best practices to follow to ensure performance, security, and scalability.
What is a RESTful API?
REST (Representational State Transfer) is style for designing networked applications. It relies on a stateless, client-server communication model where each request from a client to a server contains all the information needed to process the request. The server does not store any session state of the client between requests, making the system scalable and easy to maintain.
Key Characteristics of RESTful APIs
-
- Statelessness: The server doesn't store any information about previous requests; each request must contain all the information necessary to process it.
-
-Resource-based: Everything is a resource, which is identified by a unique URL.
-
-Uniform Interface: All interactions are done via standard HTTP methods:
-
-GET: Retrieve a resource
-
-POST: Create a new resource
-
-PUT/PATCH: Update an existing resource
-
-DELETE: Remove a resource
-
-HTTP Status Codes: Use appropriate status codes like 200 (OK), 404 (Not Found), 201 (Created), etc., to indicate the success or failure of operations.
1. Setting up a Laravel Project for API Development
-
- Start by creating a new Laravel project or use an existing one:
composer create-project --prefer-dist laravel/laravel laravel-api
-
- Laravel comes with built-in support for API routes, allowing you to define separate routing for APIs in the routes/api.php file.
-
- In the api.php file, Laravel uses the api middleware group by default, ensuring features like rate limiting are already configured.
2. Creating API Resources and Controllers
In Laravel, the resource controller pattern is used to automatically create routes for CRUD (Create, Read, Update, Delete) operations. To generate a resource controller for a model like Post, use the following Artisan command:
php artisan make:controller PostController --api
This command generates a controller with empty methods for handling the typical CRUD operations, specifically optimized for API development.
Here's an example route in routes/api.php:
Route::apiResource('posts', PostController::class);
Below are the definitions of the following routes:
-
GET /api/posts: Fetch all posts
-
GET /api/posts/{id}: Fetch a specific post
-
POST /api/posts: Create a new post
-
PUT /PATCH /api/posts/{id}: Update an existing post
-
DELETE /api/posts/{id}: Delete a post
Best Practices for RESTful API Design in Laravel
3.1 Use Appropriate HTTP Methods
Ensure that you are using the correct HTTP methods for each action:
GET: Use for retrieving data.
POST: Use for creating new resources.
PUT/PATCH: Use for updating resources.
DELETE: Use for deleting resources.
This helps maintain a clear and predictable API interface.
3.2 Return Meaningful HTTP Status Codes
Always return the appropriate HTTP status codes based on the result of the operation:
400 Bad Request: For validation errors or malformed requests.
404 Not Found: Resource is not found.
401 Unauthorized: For unauthorized access.
200 OK: For when request is successful.
201 Created: when a resource is successfully created.
500 Internal Server Error: Server-side errors.
Example in a controller method:
public function store(Request $request)
{
$post = Post::create($request->all());
return response()->json($post, 201);
}
3.3 Validation and Error Handling
Use Laravel's built-in validation to ensure the data being sent by the client is valid:
public function store(Request $request)
{
$validated = $request->validate([
'title' => 'required|string|max:255',
'content' => 'required',
]);
$post = Post::create($validated);
return response()->json($post, 201);
}
When validation fails, Laravel will automatically return a 422 Unprocessable Entity response with the validation errors.
3.4 Use API Resource Classes for Consistent Responses
Laravel's API resources provide a clean way to transform your models into JSON responses. They ensure that your API responses have a consistent structure.
Create a resource class for a model using:
php artisan make:resource PostResource
In PostResource, you can define how the response should be formatted:
public function toArray($request)
{
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->content,
'created_at' => $this->created_at->toDateTimeString(),
'updated_at' => $this->updated_at->toDateTimeString(),
];
}
To return a collection of resources:
return new PostResource($post);
3.5 Paginate Large Results
For performance reasons, large datasets should be paginated. Laravel offers a simple way to paginate results:
$posts = Post::paginate(10);
return PostResource::collection($posts);
This ensures that only a limited number of results are returned at a time, reducing the load on both the server and the client.
3.6 Versioning Your API
We may need to make changes that are not backward-compatible as your API evolves. Laravel makes API versioning easy by using route prefixes:
Route::prefix('v1')->group(function () {
Route::apiResource('posts', PostController::class);
});
This allows you to maintain multiple versions of your API (e.g., v1, v2), ensuring that old clients continue to work with older versions of the API.
3.7 Rate Limiting
To protect your API from abuse, you should implement rate limiting. Laravel includes rate limiting middleware by default. You can adjust rate limiting in app/Http/Kernel.php:
protected $middlewareGroups = [
'api' => [
'throttle:60,1', // 60 requests per minute
],
];
This configuration limits clients to 60 requests per minute, preventing excessive load on your API.
3.8 Authentication and Authorization
For API authentication, Laravel provides tools like Sanctum and Passport.
Laravel Sanctum is able to authenticate API requests via tokens:
Install Sanctum:
composer require laravel/sanctum
php artisan migrate
Add the Sanctum middleware to api.php:
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
With this setup, clients must send a token with each request to access protected resources.
4. Handling CORS (Cross-Origin Resource Sharing)
If your API is consumed by clients from different origins (e.g., a mobile app or another web domain), you'll need to configure CORS.
Laravel includes a middleware to handle CORS in the app/Http/Middleware directory. You can setup the CORS settings in config/cors.php.
For instance, you can allow specific domains to access your API:
'allowed_origins' => ['https://example.com'],
This ensures that only requests from certain origins are allowed, protecting your API from unauthorized use.
Summary
By following best practices such as using appropriate HTTP methods, returning meaningful status codes, implementing validation, and securing your API with authentication and rate limiting, you can ensure that your API is easy to maintain, scalable and secure.
By using Laravel's features like API resources, pagination, Sanctum, and CORS configuration, you can create robust and flexible APIs that provide smooth integration with different platforms and clients.