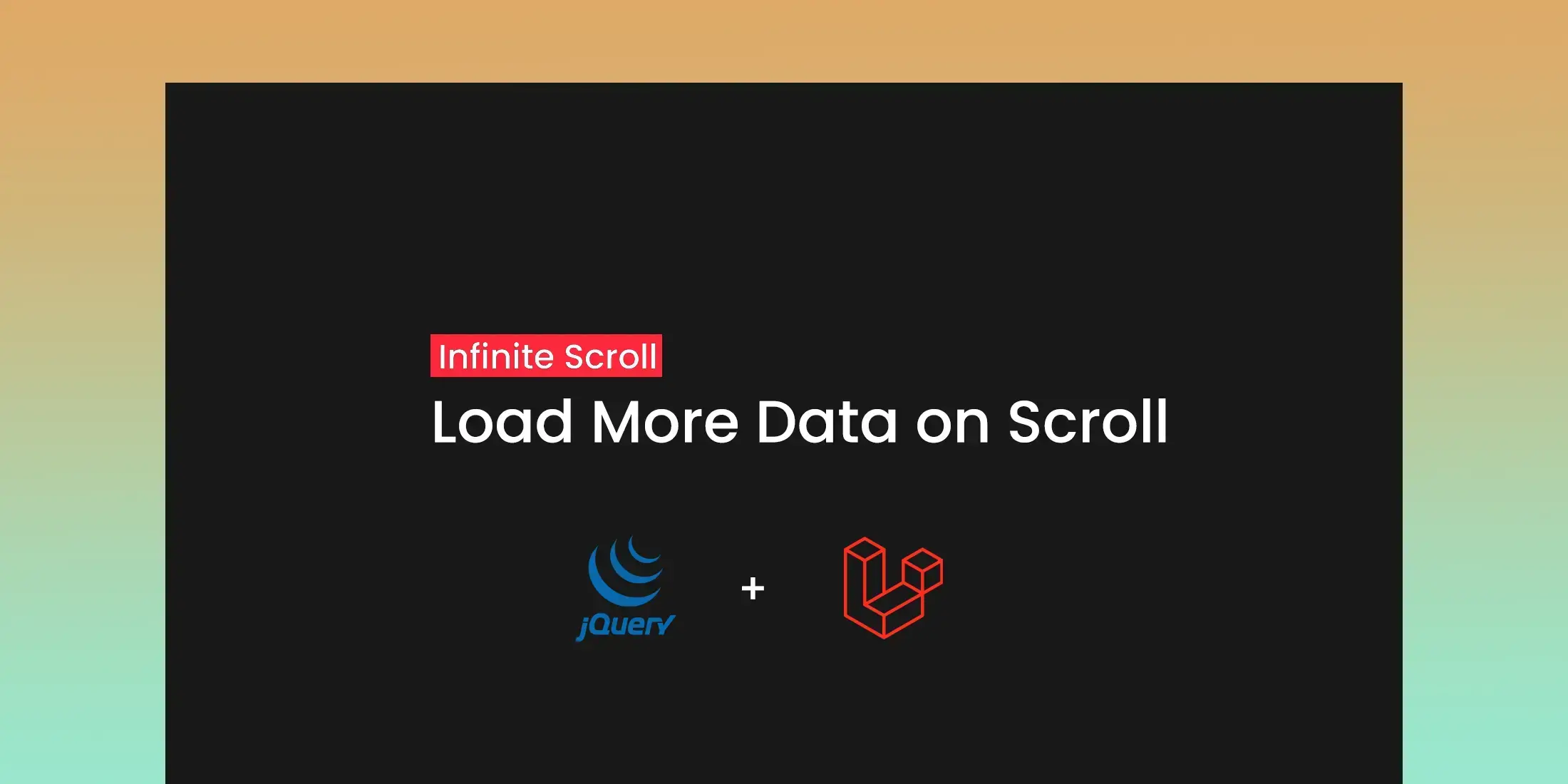
Infinite Scroll in Laravel Using jQuery and AJAX
Infinite scroll is a popular technique that automatically loads more content as users reach the bottom of the page.By using this Infinite scroll technique in Laravel we don't need to implement pagination.
We will use jQuery and AJAX,to send the request, it prevents full page reload resulting in faster loading times.
Setting Up Laravel and Controller and Route
let's set up the Laravel controller and route for handling the infinite scroll requests. In your controller, add a method to fetch and paginate posts:
public function infiniteScroll()
{
$posts = Post::orderBy('created_at', 'desc')->paginate(20);
if (request()->ajax()) {
return response()->json([
'posts' => view('website.posts-ajax', compact('posts'))->render(),
'next_page' => $posts->currentPage() + 1,
'last_page' => $posts->lastPage()
]);
}
return view('website.post', compact('posts'));
}
Next, define a route to handle the infinite scroll requests:
Route::get('/posts', [PostController::class, 'infiniteScroll']);
Partial View for AJAX Response
Create a partial view (resources/views/website/posts-ajax.blade.php) to render posts loaded via AJAX:
<?php foreach ($posts as $post): ?>
<div class="post">
<h2>{{ $post->title }}</h2>
<p>{{ $post->created_at }}</p>
</div>
<?php endforeach; ?>
Implementing jQuery for Infinite Scroll
Set up a Blade view (resources/views/website/post.blade.php) to display the initial posts and prepare for infinite scroll:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>Infinite Scroll - Elegant Laravel</title>
<link rel="preconnect" href="https://fonts.bunny.net">
<link href="https://fonts.bunny.net/css?family=figtree:400,600&display=swap" rel="stylesheet" />
</head>
<body>
<div class="post-container">
@foreach ($posts as $post)
<div class="post">
<h2>{{ $post->title }}</h2>
<p>{{ $post->created_at }}</p>
</div>
@endforeach
</div>
<div class="loading" style="display: none;">
<p>Loading more posts...</p>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
var page = 1;
var lastPage = {{ $posts->lastPage() }};
$(window).scroll(function() {
if ($(window).scrollTop() + $(window).height() >= $(document).height() - 100) {
page++;
if (page <= lastPage) {
loadMoreData(page);
}
}
});
function loadMoreData(page) {
$.ajax({
url: '/posts?page=' + page,
type: "get",
beforeSend: function() {
$('.loading').show();
}
}).done(function(data) {
if (data.posts == "") {
$('.loading').html("No more records found");
return;
}
$('.loading').hide();
$(".post-container").append(data.posts);
lastPage = data.last_page;
}).fail(function(jqXHR, ajaxOptions, thrownError) {
console.error('Server not responding...', thrownError);
});
}
});
</script>
</body>
</html>