
Per-Second Rate Limiting in Laravel 11
Per-Second Rate Limiting is a new feature in Laravel 11, providing more control over the rate limiting of your application's routes.For instance, you might want to ensure that a user can't make more than one request per second to avoid overloading your server.
Setting Up Per-Second Rate Limiting
You can define rate limits for your routes on a per-second basis, in addition to the traditional per-minute rate limits. This feature is beneficial for APIs that require stricter rate limits to prevent misuse.By setting limits on a per-second basis, you can protect your server from rapid bursts of traffic that might not breach per-minute limits but can still cause significant load and potential downtime.
Define Rate Limits in RouteServiceProvider
To set up per-second rate limiting, you need to define custom rate limits in the RouteServiceProvider. This is done in the boot method of your RouteServiceProvider.
Apply Rate Limits to Routes
After defining the rate limits, you can apply them to specific routes using middleware. In your 'routes/api.php', define a route that uses the rate limiting middleware:
In this example, the /test route is limited to 1 request per second and 10 requests per minute.
Output
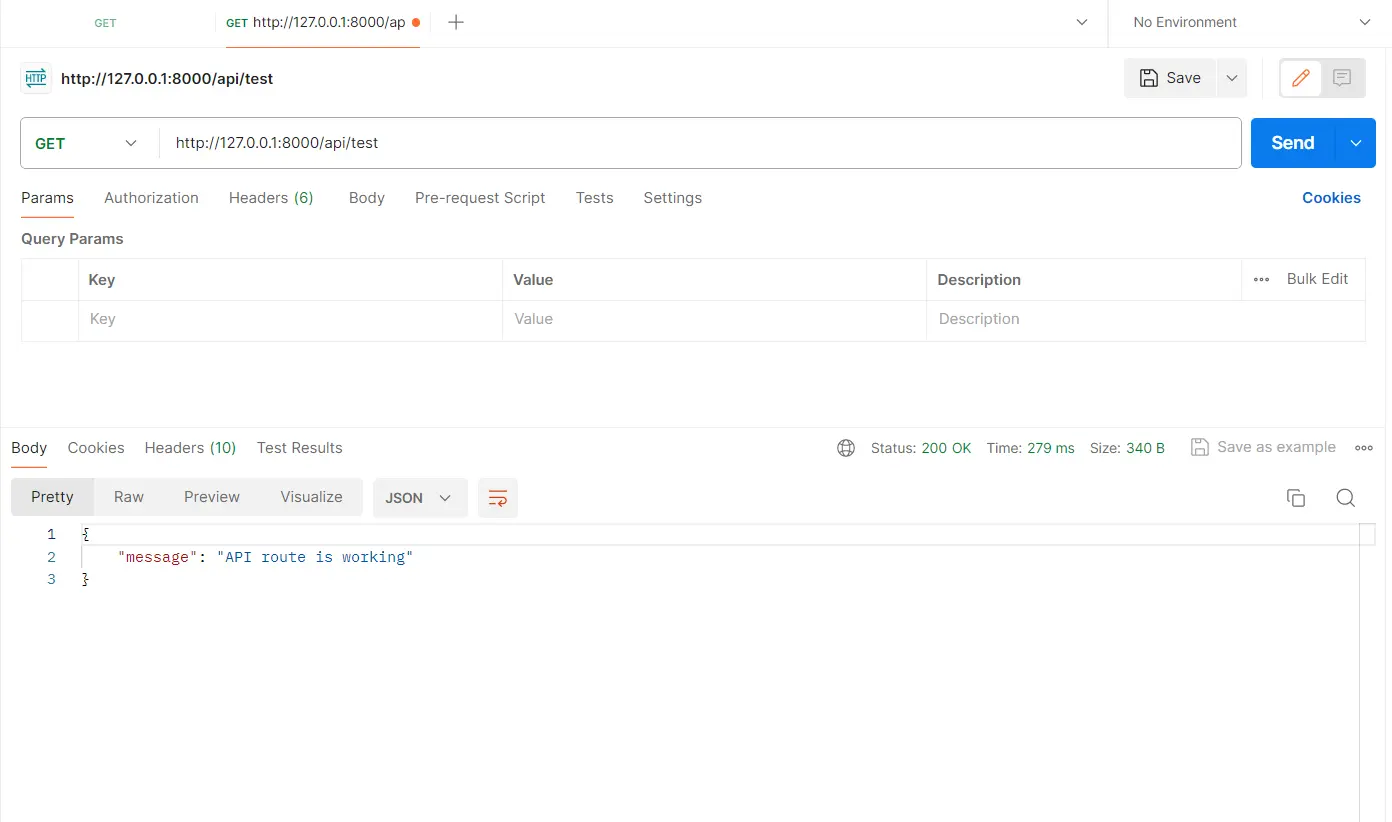
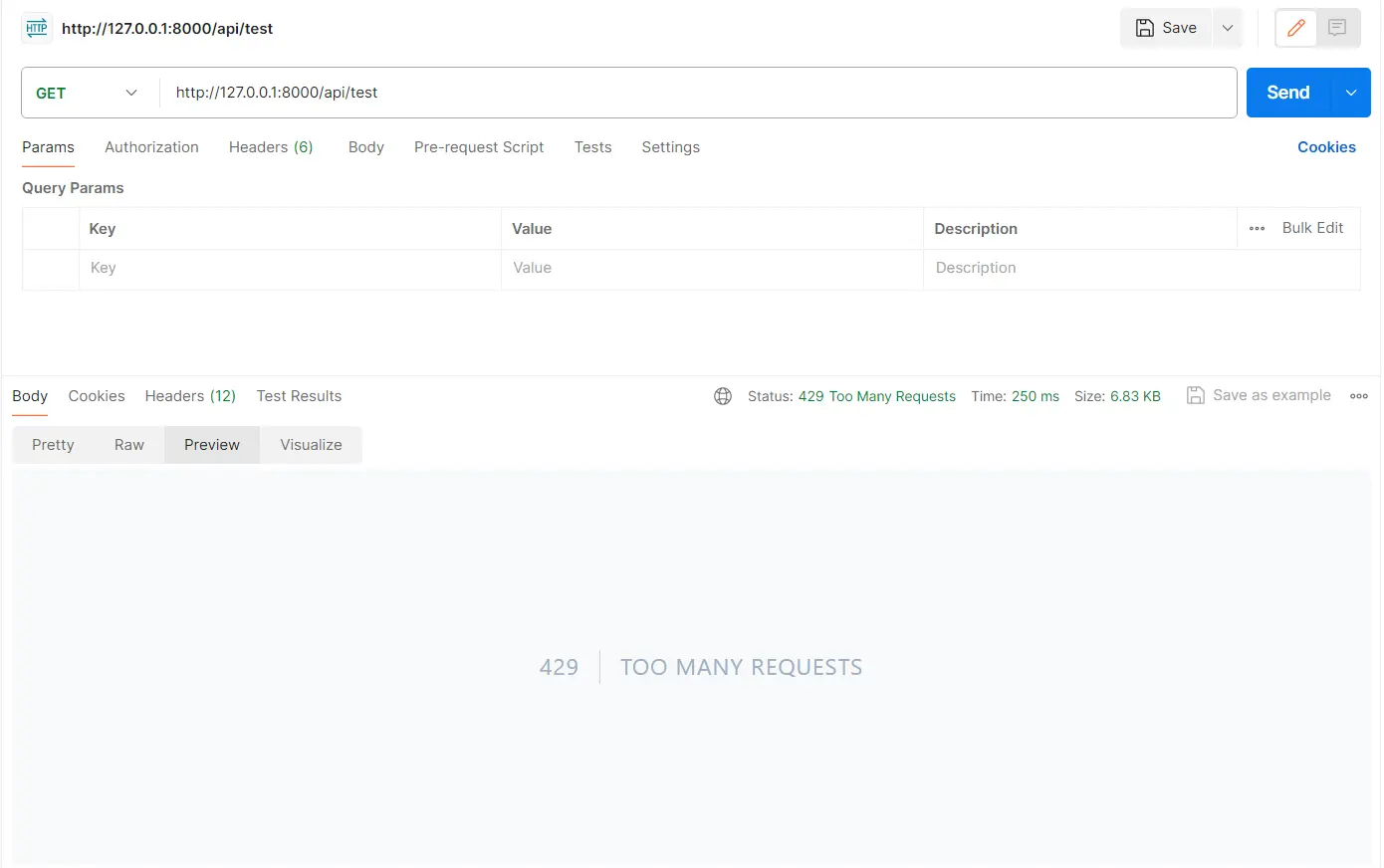
Benefits of Per-Second Rate Limiting
Enhanced Performance: By limiting requests on a per-second basis, you can prevent server overload caused by rapid bursts of traffic.
Improved Security: Stricter rate limits help mitigate abuse and potential DoS attacks.