
How to Use Lateral Joins in Laravel for Fetching Limited Records
In web applications, effective data retrieval is crucial for performance and user experience. Traditional SQL joins work well in many scenarios, but they often struggle when limiting the number of related records. This is where lateral joins come into play. lateral joins enable you to execute subqueries for each row returned by the outer query. In this article, we will explore how to implement lateral joins in Laravel to efficiently fetch related data.
Suppose you have users and orders tables.We have user_id in orders table.So,if we want to get the 3 orders of every user, you can’t do it with joins or the relationship.There is no way to add limit on it .Here lateral join comes to play his role.It accepts two arguments the subquery and its table alias.
Note : Lateral joins are currently supported by PostgreSQL, MySQL >= 8.0.14, and SQL Server.
Reference : Laravel Official Documentation
Example
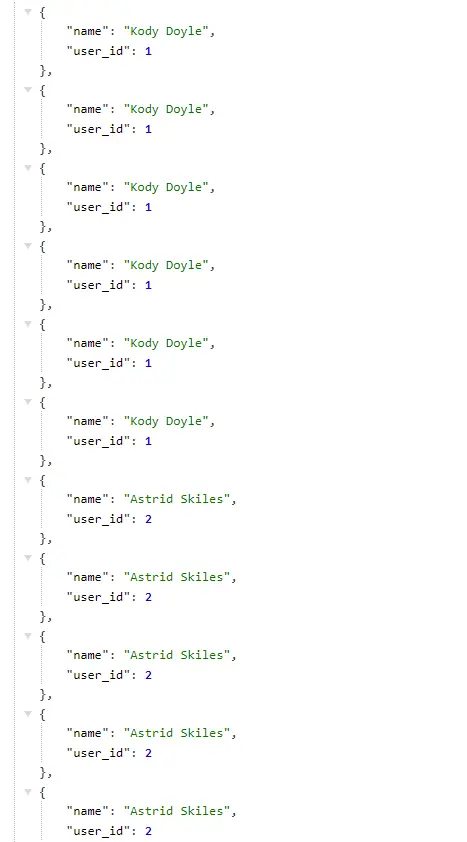
Understanding Lateral Joins
Lateral joins allow subqueries to reference columns from the outer query, making it possible to limit results per group effectively. For instance, if you want to fetch the latest three orders for each user from the users and orders tables, traditional joins would be inadequate. Here's how lateral joins address this limitation.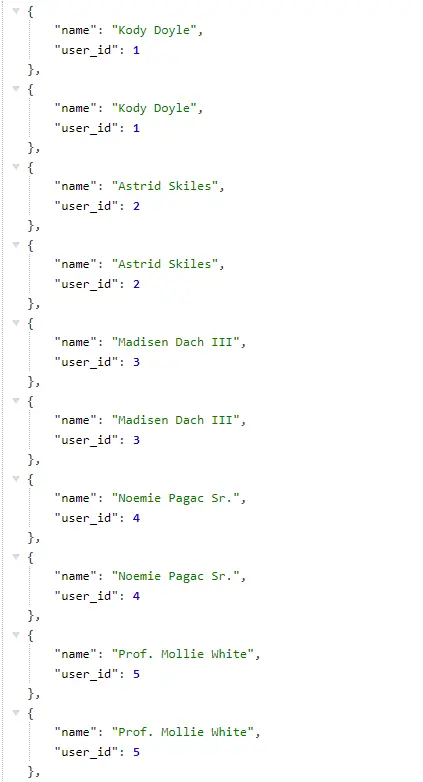
Benefits of Using Lateral Joins
Efficiency: Lateral joins reduce the complexity of fetching limited records for related data, improving query performance.
Flexibility: They allow for more complex queries that traditional joins cannot handle, especially in aggregating and limiting records.
Database Compatibility: Lateral joins are supported by modern database systems like PostgreSQL, MySQL 8.0.14+, and SQL Server, making them a viable option for various applications.
Considerations
While lateral joins offer significant advantages, they should be used judiciously:
Database Support: Ensure your database version supports lateral joins before implementation.
Performance Monitoring: Regularly monitor query performance, as complex joins can sometimes lead to slower execution times if not optimized properly.