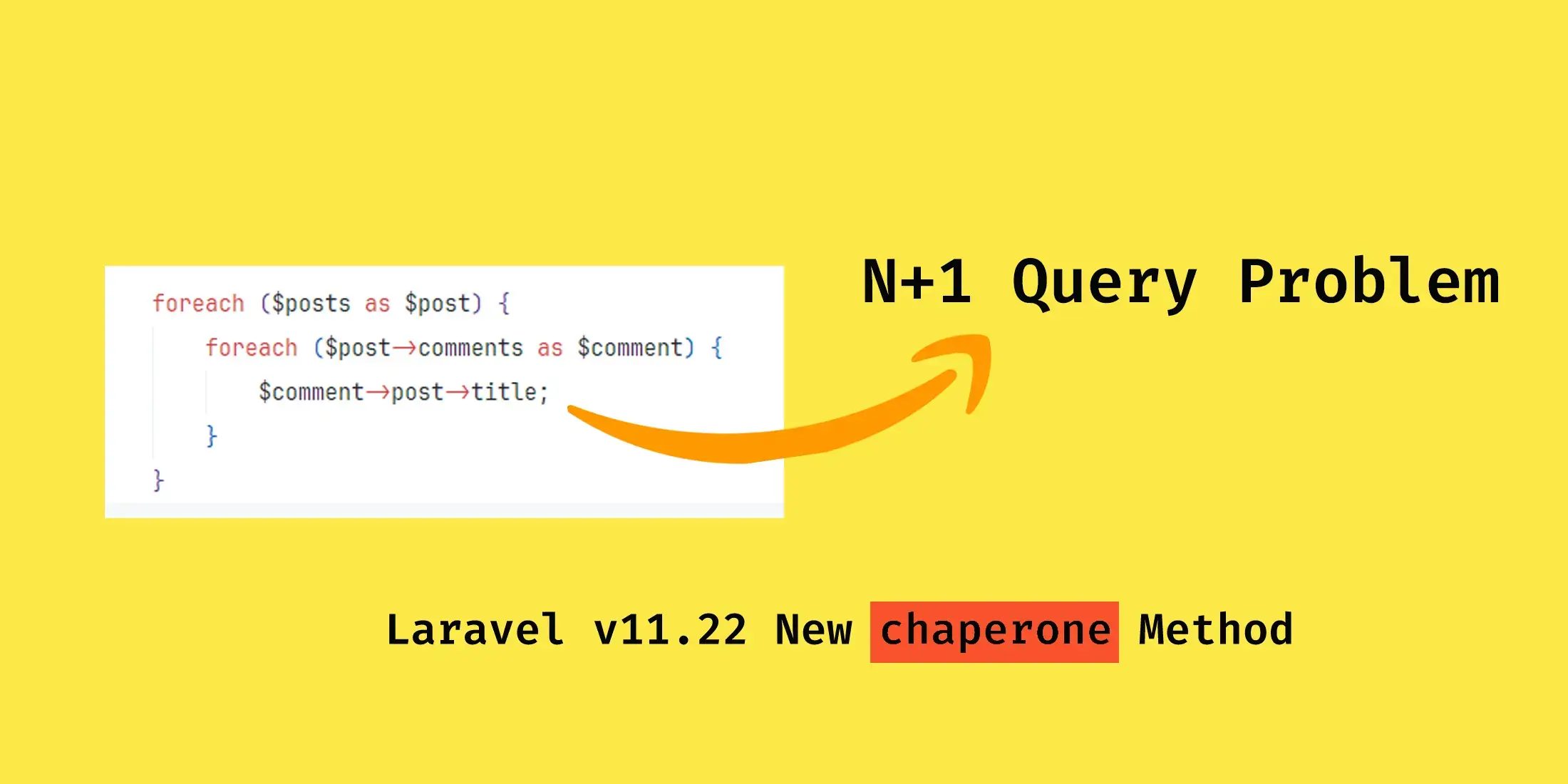
How to Solve the N+1 Query Problem Using Eager Loading in Laravel 11 with chaperone Method
Introduction
The N+1 query is a common problem that can impact the performance of Laravel applications. It occurs when your application executes one query to retrieve the parent model and additional queries for each related model. Which leads to potentially hundreds or thousands of queries. To address this, Laravel 11.22 introduced a new method called chaperone that provides a solution to the N+1 problem.
You can use the chaperone method to optimize your queries and prevent the N+1 issue. We'll use a practical example involving a Post model with related Comment models to explain the problem and its solution.
Understanding the N+1 Query Problem
Imagine a blog application where each post can have multiple comments. You might retrieve posts along with their comments like this by using the following code:
At first glance, this code seems fine. ituses eager loading (eager loading loads all of its content) to fetch the comments relationship. But, if you look closely, each time you access $comment->post, an additional query is executed to fetch the post of that comment. If you have 100 posts, and each post has 10 comments, this results in 101 queries (1 for posts, 1 for comments, and 100 for post), leading to the N+1 query problem.
AS you can see in the output, there is so many queries.
Introducing the chaperone Method
Laravel 11.22 introduces the chaperone method, which can be applied to relationships to ensure that all related models are loaded in the most efficient way possible, solving the N+1 query problem.
let use the above example again of a blog application where each post can have multiple comments using the chaperone method by the following code:
By applying the chaperone method to the comments relationship, Laravel optimizes the query so that the related Post models are eager-loaded (eager loadingloads all of its content) alongside the comments. This greatly reduces the number of queries executed.
Implementing the chaperone Method in the Route
Now that we have added the chaperone method to the relationship, we can see how it improves our route by using the following code:
In the above example Laravel fetches all the necessary data in a single query, greatly improving performance by eliminating the N+1 query problem.
After using the chaperone method, the number of queries has been decreased drastically.
Alternative Approach
If you prefer not to modify the relationship method, you can also apply the chaperone method directly within the eager loading(eager loadingloads all of its content) definition by using the following code:
In the above example, the chaperone method is used directly in the with clause, which also ensures that the N+1 query problem is resolved without altering the Post model itself.
In some cases, you may want to get the fresh instance which lead to n+1 query problem then you can follow that way too but there is also another method name withoutChaperone
Conclusion
The N+1 query is a common problem that can impact the performance of Laravel applications. It occurs when your application executes one query to retrieve the parent model and additional queries for each related model. Which leads to potentially hundreds or thousands of queries. To address this, Laravel 11.22 introduced a new method called chaperone that provides a solution to the N+1 problem.
Whether you choose to apply chaperone directly on the relationship or within the with clause , this method will ensure that your application performs efficiently, even as it scales. By using chaperone , you can focus on building powerful applications without worrying about the N+1 query problem.