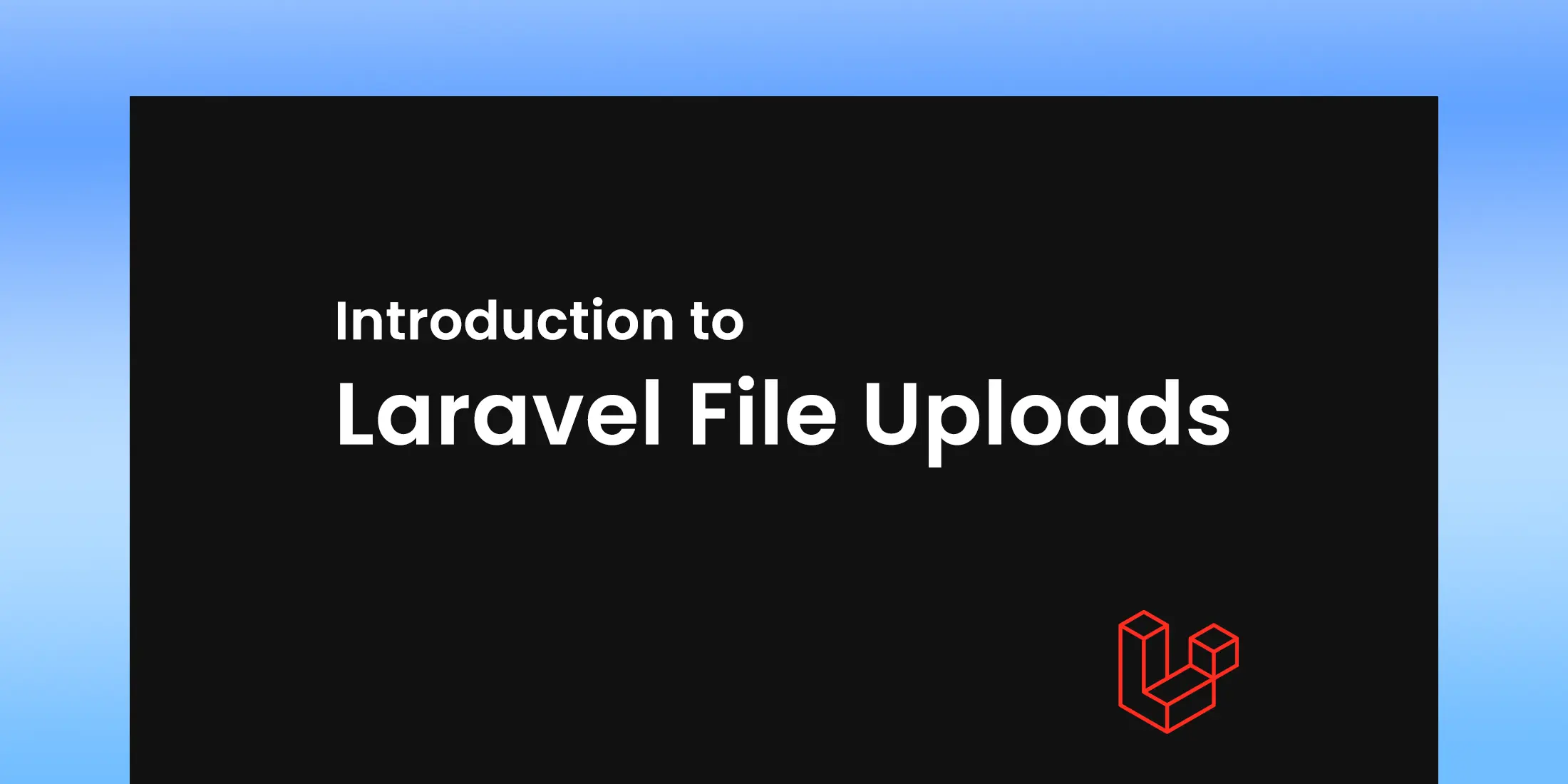
Introduction to Laravel File Uploads
1. Introduction to Laravel File Uploads
Laravel simplifies the file upload process using its powerful request and filesystem abstractions. When a file is uploaded, Laravel treats it as an instance of the UploadedFile class, which provides helpful methods for handling uploaded files.
A typical form for uploading files looks like this in Blade:
The enctype="multipart/form-data" attribute ensures that file data is correctly transmitted to the server.
2. File Validation for Security
Before storing files, it's crucial to validate them to prevent malicious uploads or incorrect file types. Laravel provides robust validation rules to ensure only specific file types, sizes, or formats are accepted.
Example of File Validation:
In this case:
- The mimes rule ensures that only certain file types (JPEG, PNG, JPG, GIF) are allowed.
- The max rule restricts the file size to 2MB.
Other Useful Validation Rules:
- file: Ensures the uploaded input is a file.
- image: Restricts the upload to image files (JPG, PNG, BMP, GIF, etc.).
- mimetypes: Validates the file based on its MIME type (like text/plain, application/pdf).
Validation helps protect your system from potential security threats, like uploading executable files or excessively large files.
3. Storing Files in Laravel
Once validated, the file can be stored using the store() method. By default, this method stores files in the local file system, which refers to the storage/app directory.
Example:
Here, the file will be stored in the storage/app/images directory. The store() method automatically generates a unique filename to avoid conflicts.
Customizing the File Path
You can specify a custom storage location using the second argument of the store() method:
In this case, the file will be stored in storage/app/public/uploads.
4. File Storage Configuration (Local and Cloud)
Laravel’s file storage system is highly customizable, and you can configure it to use local storage or a cloud service like Amazon S3. Laravel supports several storage drivers, which are set in the config/filesystems.php file.
Local Storage Configuration
By default, Laravel uses the local storage driver. To make files publicly accessible, you need to create a symbolic link from public/storage to storage/app/public. You can create this link using the Artisan command:
This command makes files stored in storage/app/public accessible from the web.
Cloud Storage Configuration
To use cloud storage like Amazon S3, you’ll need to install the AWS SDK for PHP:
After that, configure the S3 driver in your .env file:
Then, you can store files in S3 like this:
Similarly, you can configure Google Cloud Storage or Microsoft Azure by adding the respective drivers and credentials in the configuration file.
5. Retrieving and Managing Uploaded Files
Once files are uploaded, you can retrieve and manage them using Laravel’s storage facade. Laravel makes it easy to access files, check if a file exists, or delete files.
Accessing Files
To retrieve the URL of a file:
This will generate a publicly accessible URL for files stored using the public disk.
Checking if a File Exists
Deleting Files
This will delete the file from the storage directory.
6. Securing File Uploads
File uploads can pose security risks if not handled properly. Here are some best practices to secure file uploads in Laravel:
1. Validate File Types and Sizes:
As mentioned earlier, always validate the file type and size to prevent malicious uploads.
2. Use Secure Directories:
Store uploaded files in directories outside the public folder (like storage/app) to prevent direct access. Only serve files after proper authentication.
3. Sanitize File Names:
While Laravel’s store() method automatically generates safe file names, if you’re manually naming files, ensure the names are sanitized to avoid vulnerabilities like path traversal.
4. Limit File Permissions:
Set correct permissions for uploaded files. For example, don’t give executable permissions to files stored on the server.
7. Displaying and Downloading Files
Laravel makes it simple to display or download uploaded files in your application.
Displaying Images
You can directly display images stored in the public disk by accessing their URL:
If you're storing files in private storage, you'll need to generate a secure URL and serve the file using controllers.
Downloading Files
To allow users to download files, you can use the download() method:
This method generates a response that triggers the download of the specified file.
8. Best Practices for File Uploads and Storage in Laravel
Here are some best practices to follow when handling file uploads in Laravel:
1. Use the Appropriate Storage Driver
Choose the storage driver based on your project needs. For simple projects, the local disk may suffice, but for large-scale applications, consider using cloud storage like Amazon S3 or Google Cloud for scalability and reliability.
2. Store Files Outside the Public Directory
Always store sensitive or private files outside the public directory. Serve them securely through routes or controllers after proper authentication.
3. Regularly Clean Up Unused Files
Ensure that files that are no longer needed (such as expired uploads) are regularly deleted to free up space and improve application performance.
4. Optimize File Sizes
Compress images or large files before storing them. This reduces storage space and improves the application’s performance, especially when serving files to end users.
5. Securely Serve Private Files
When dealing with private or sensitive files, use signed URLs or check for user authentication before allowing access to the files.
Conclusion
Handling file uploads and storage is a common requirement in web development, and Laravel simplifies this process through its robust filesystem and storage mechanisms. Whether you’re working with local storage or cloud services like Amazon S3, Laravel provides the flexibility and security you need to handle file uploads efficiently.
By following the best practices outlined in this guide, you can ensure that your application is secure, scalable, and efficient when dealing with file uploads and storage.
Key Takeaways:
- Laravel makes file uploads easy with the store() method.
- Use proper file validation rules to ensure safe uploads.
- Choose the appropriate storage driver (local or cloud) based on your needs.
- Securely store and manage files using Laravel’s Storage facade.
- Follow best practices like securing file uploads and optimizing file sizes.
By mastering these concepts, you can build robust and secure applications that handle file uploads with ease!